The Laravel Built-In Client allows you to connect with External APIs (r)
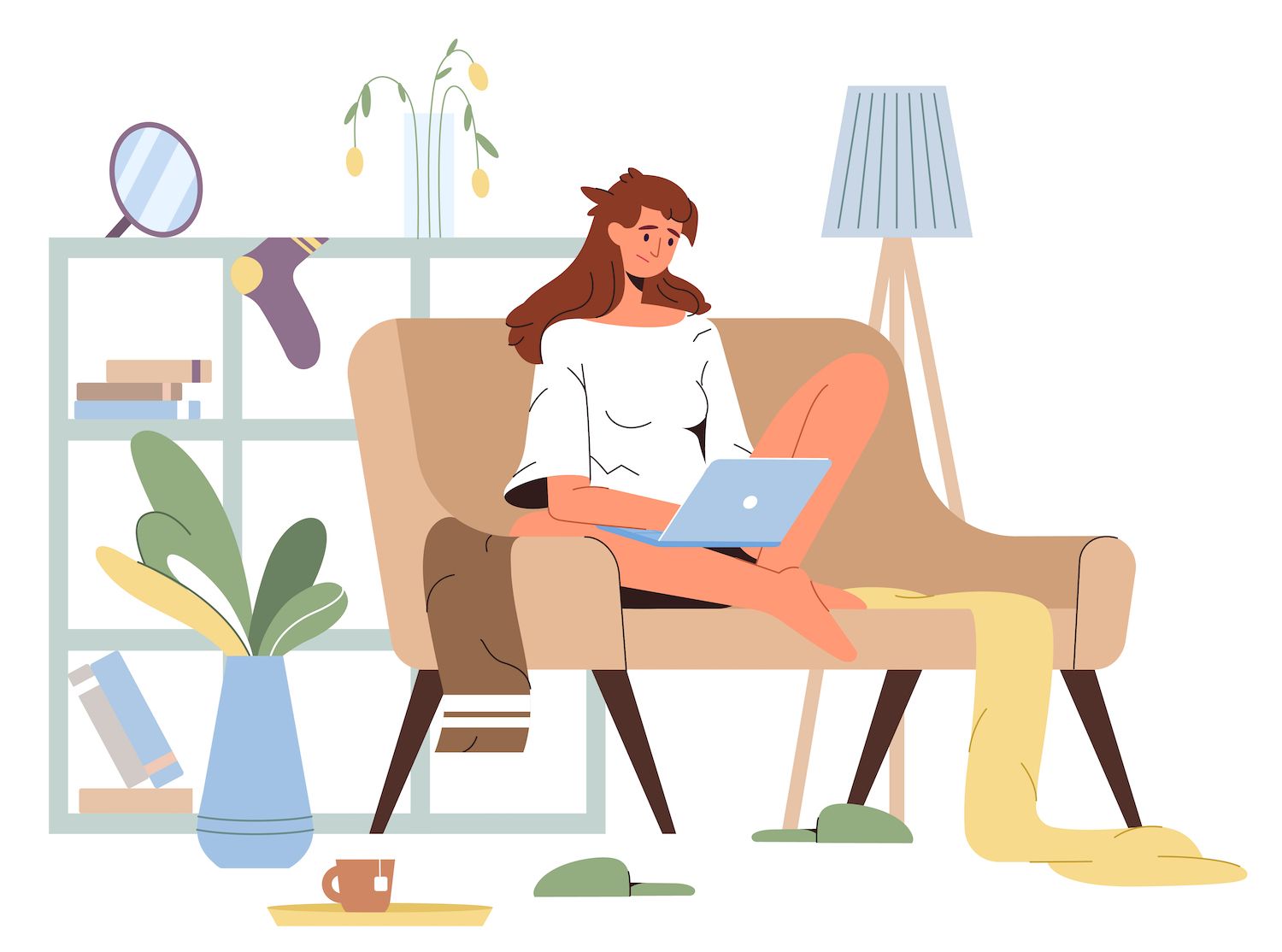
Share this on
This article will discuss the ways to utilize the Laravel HTTP client to submit requests, examine responses, create middleware and macros, as well as more.
Laravel HTTP Client takes care of the heavy lifting for APIs
Guzzle is a simple HTTP client built to work with PHP. Guzzle is able to handle various types of requests like the request types GET
, POST
, PUT
, and DELETE
as well as streaming capabilities as well as multipart request. By using the Guzzle HTTP client making either synchronous or asynchronous requests to servers is feasible. Additionally, it includes an excellent middleware program that can modify the way the client behaves.
The Laravel HTTP client wrapper built on Guzzle but it comes with additional functions. It supports the ability unsuccessful requests to be re-tried, as well as some helper applications that utilize JSON information. A majority of functions in Laravel HTTP clients are similar to Guzzle.
The prerequisites
In the following sections we'll give more details on the Laravel HTTP client. To follow the tutorial it will require:
- Composer and PHP both are on the computer
- Postman
How To Make Requests
If you want to know how you can use an HTTP client to make an request, utilize a wide range of hosted APIs, for instance such as ReqRes.
Begin by installing the HTTP package while you're making the application. Inside the App/Http/Controllers/UserController.php file, add the following code, starting with the use statement at the beginning of the file and the remaining code inside the index function.
use Illuminate\Support\Facades\Http; return Http::get("https://reqres.in/api/users?page=2");
Note: For complex use cases, you can also transmit the request using headers by using the method withHeaders. usingHeaders
method.
Within the same file, create an entirely new file by applying this code:
function post() $response = Http::withHeaders([ 'Content-Type' => 'application/json', ])->post('https://reqres.in/api/users', [ 'name' => 'morpheus', 'job' => 'leader', ]); return $response;
Create a new route within your routes/web.phpfile:
Route::get('post',[UserController::class,'post']);
In the meantime, Postman can be used to verify this method. Open Postman and add http://127.0.0.1:8000/post as the URL, with the type of request as GET
. Once you press send and receive the following message:
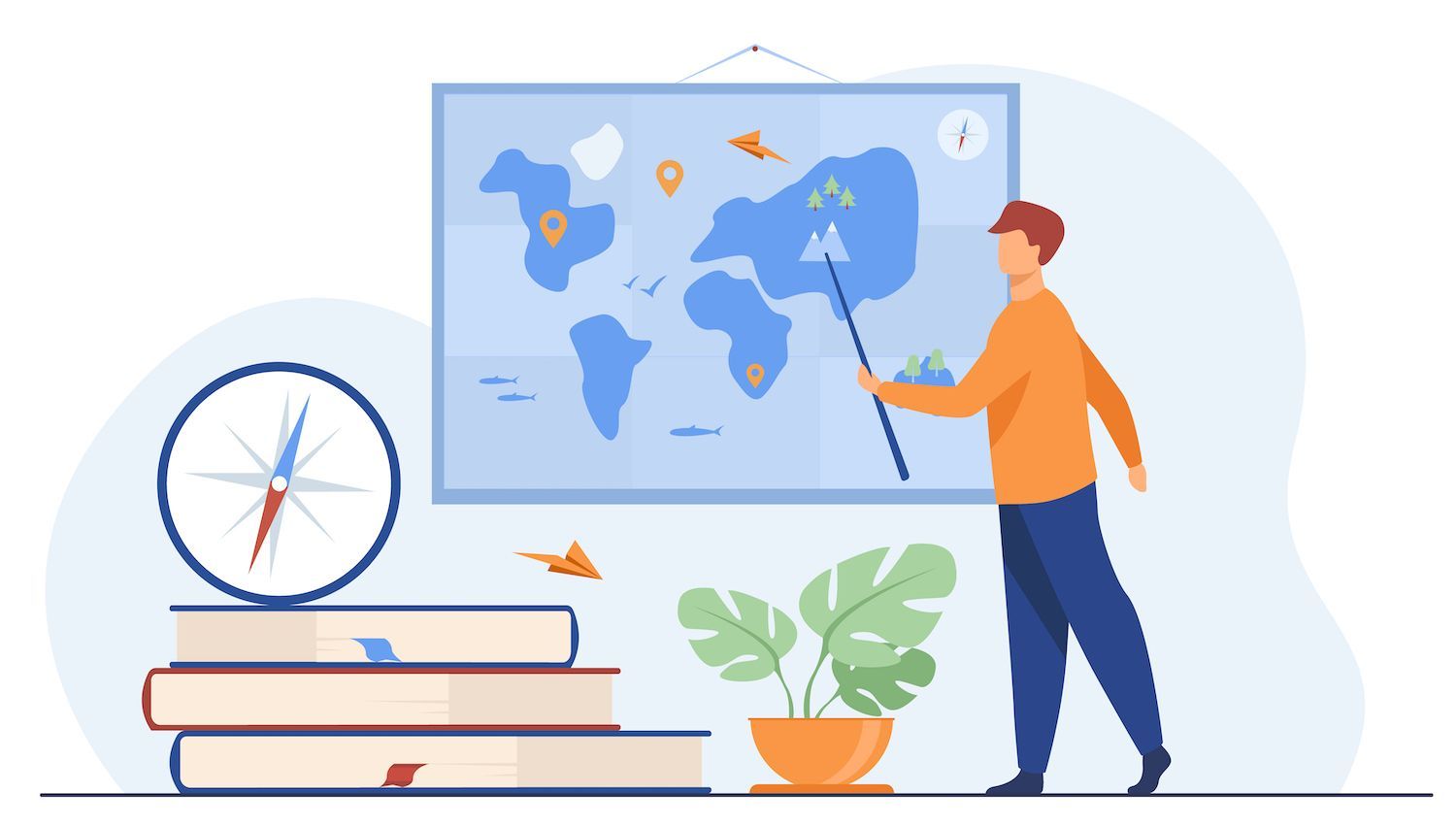
Concurrent Demands
The performance of parallel requests is significantly improved since you're able to access more data in the same time. Laravel's HTTP client permits you to make simultaneous requests using the pool method.
Inside App/Http/Controllers/UserController.php, add the following code:
use Illuminate\Http\Client\Pool; function concurrent() $responses = Http::pool(fn (Pool $pool) => [ $pool->get('https://reqres.in/api/users?page=2'), $pool->get('https://reqres.in/api/users/2'), $pool->get('https://reqres.in/api/users?page=2'), ]); return $responses[0]->ok() && $responses[1]->ok() && $responses[2]->ok();
After that, you can include the route in your routes/web.phpfile.
Route::get('concurrent',[UserController::class,'concurrent']);
This message every time you access the site:
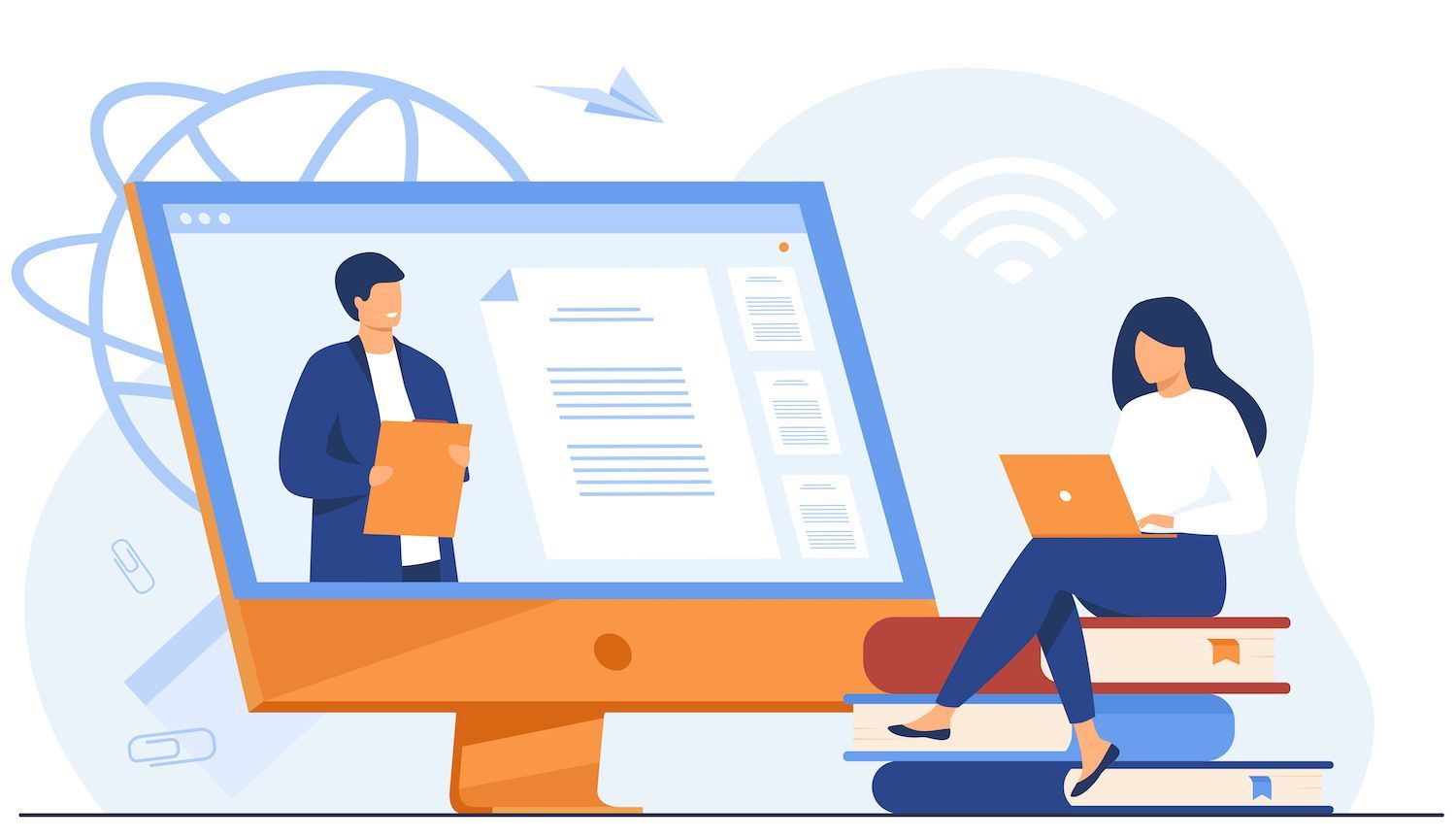
Macros Request Macros
Request macros are useful in interacting with the common API routes.
To create the macro, you need to define the macro inside the boot method of the app/Http/Providers/AppServiceProvider.php file using the code below:
use Illuminate\Support\Facades\Http; Http::macro('reqres', function () return Http::baseUrl('https://reqres.in/api'); );
Note: Make sure to include the usage statement on the very first line of your file.
You can then apply the macro within the UserController
to include the following code:
function macro() $response = Http::reqres()->get('/users?page=2'); return $response;
It is evident that the macro is there, you do not need to enter the URL in full each time.
In the final step, you may create a new route within your routes/web.php file using the following code:
Route::get('macro',[UserController::class,'macro']);
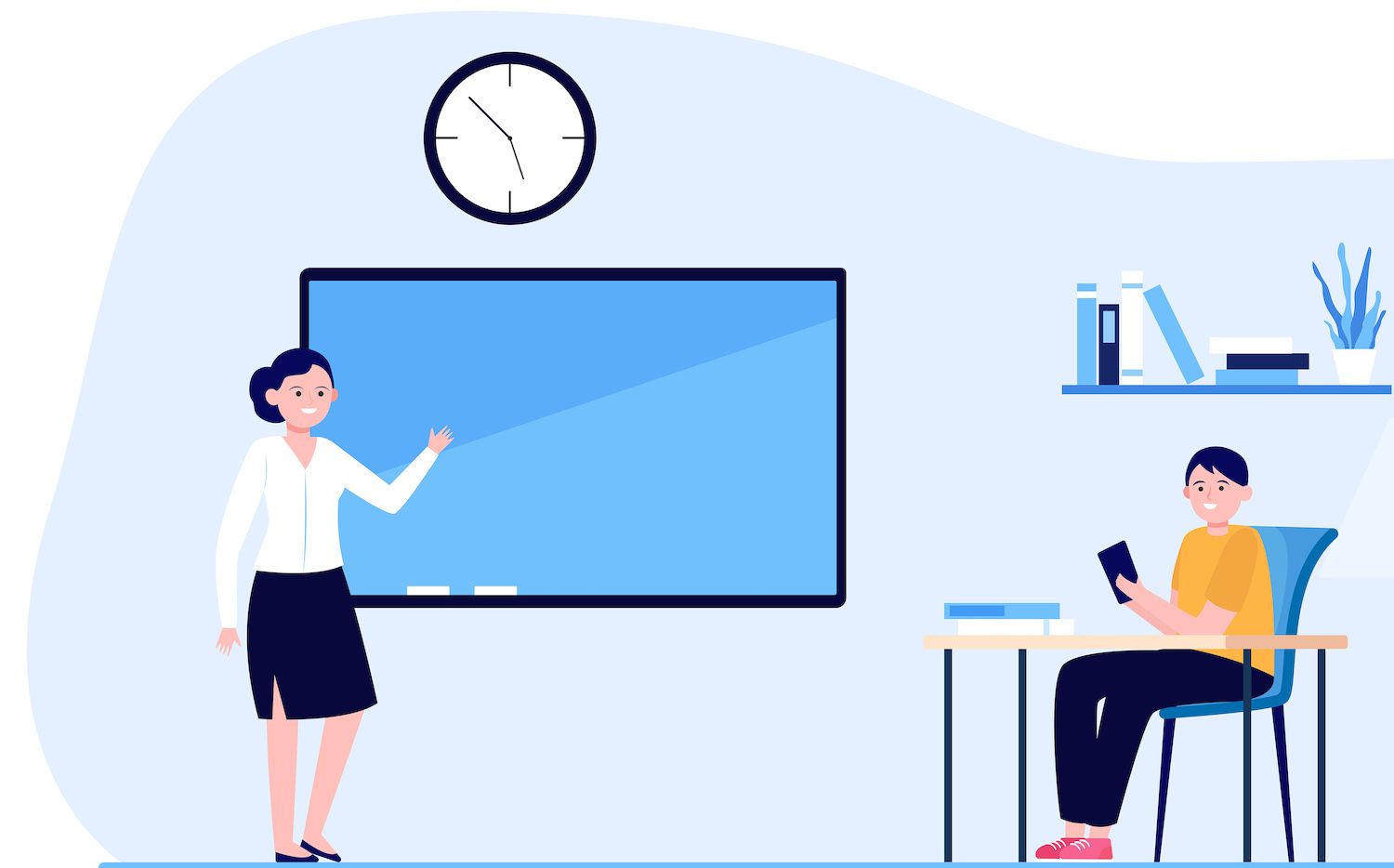
What is the best way to decode responses
In order to decode the response and ensure the API request was legitimate, you can utilize the status option in the client. The method is used to retrieve the status message by the server and present the status message.
To test this out, replace the previous macro code with the code below inside the App/Http/Controllers/UserController.php file:
function macro() $response = Http::reqres()->get('/users?page=2'); return $response->status();
The status code 200 signifies that the request was completed successfully.
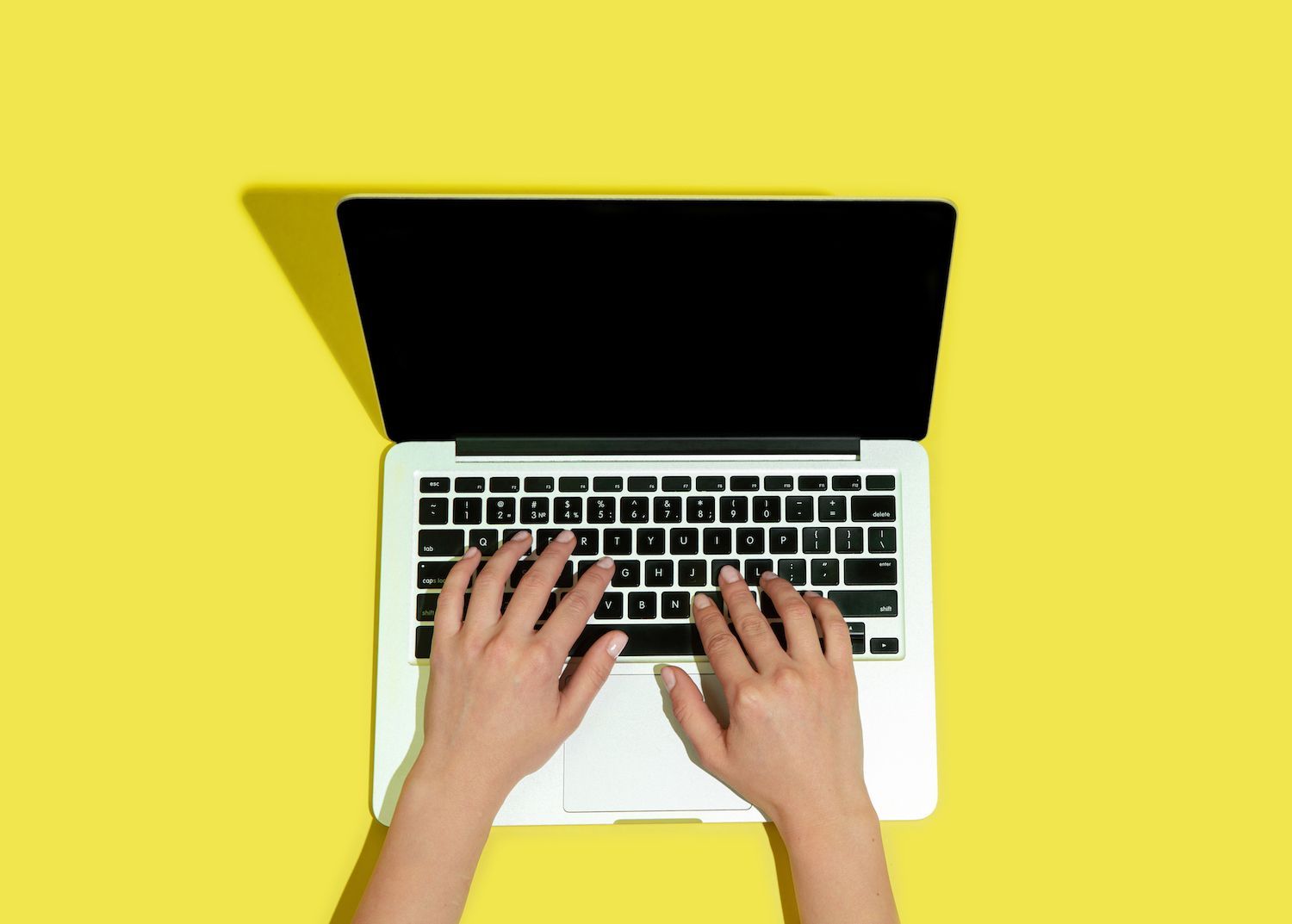
What is the best way to evaluate JSON APIs?
Laravel provides several tools that allow you to examine the JSON APIs as well as their replies. The helper functions include JSON, GetJson, PostJson putJson, patchJson. deleteJson, and so on.
To better understand Testing better, create a test scenario that follows this the GET
user's journey. When you bootstrap the Laravel application then the test example has already been created. Inside the tests/Feature/ExampleTest.php file, replace the existing code with the following:
getJson('/users'); $response->assertStatus(200);
The extra code pulls the JSON information from the place of origin and checks if the status code is 200 or not.
After adding the test code, execute this command inside your terminal, in order to execute the tests:
./vendor/bin/phpunit
After the test is completed after the tests are completed and you'll see tests that were conducted twice and both were successful.
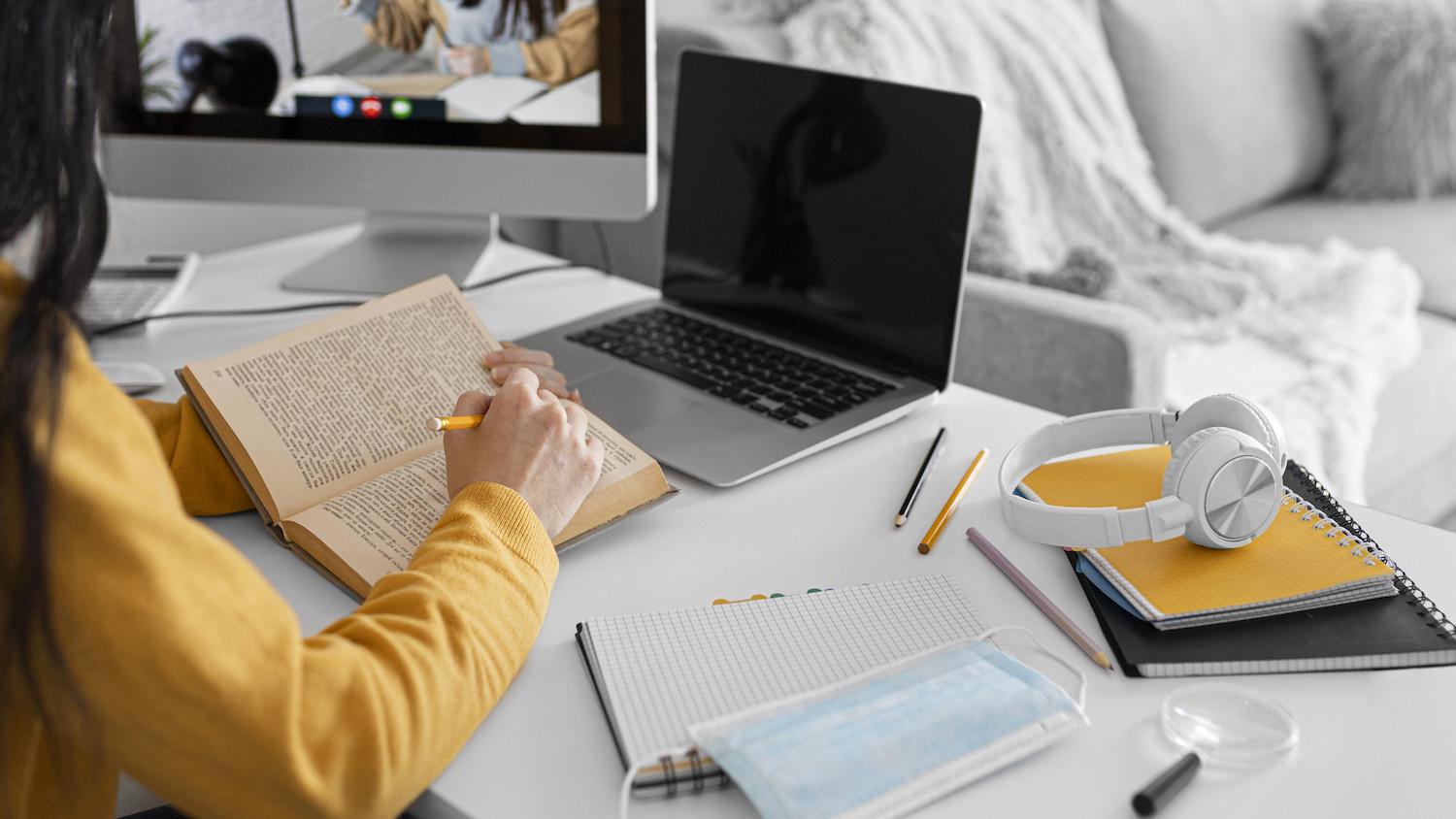
Similar to that it is possible to test for different types of inquiries as well as employ additional helper techniques to conduct more advanced testing.
What to do when faced with events
- RequestSending that is prior to the time that the request will be sent.
- ResponseReceived This is the time when a response is received.
- ConnectionFailed that is, the case when there is no response.
All three events include the $request
property to inspect the Illuminate\Http\Client\Request
instance, and ResponseReceived
has an additional $response property
. These properties are very useful for taking actions in the aftermath of the event. For example, you might require sending an email in response to an event that has been successful in responding.
To create an event and listener, navigate to the app/Providers/EventServiceProvider.php file and replace the listen array with the following code.
protected $listen = [ Registered::class => [ SendEmailVerificationNotification::class, ], 'Illuminate\Http\Client\Events\ResponseReceived' => [ 'App\Listeners\LogResponseReceived', ], ];
Run the following command on your terminal
php artisan event:generate
The above command will create the app/Listeners/LogResponseReceived.php listener. Replace the code from this file with the instructions:
info($response->status()); /** * Handle the event. * * @param \Illuminate\Http\Client\Events\ResponseReceived $event * @return void */ public function handle(ResponseReceived $event)
The information log that contains the status code is displayed within the terminal.
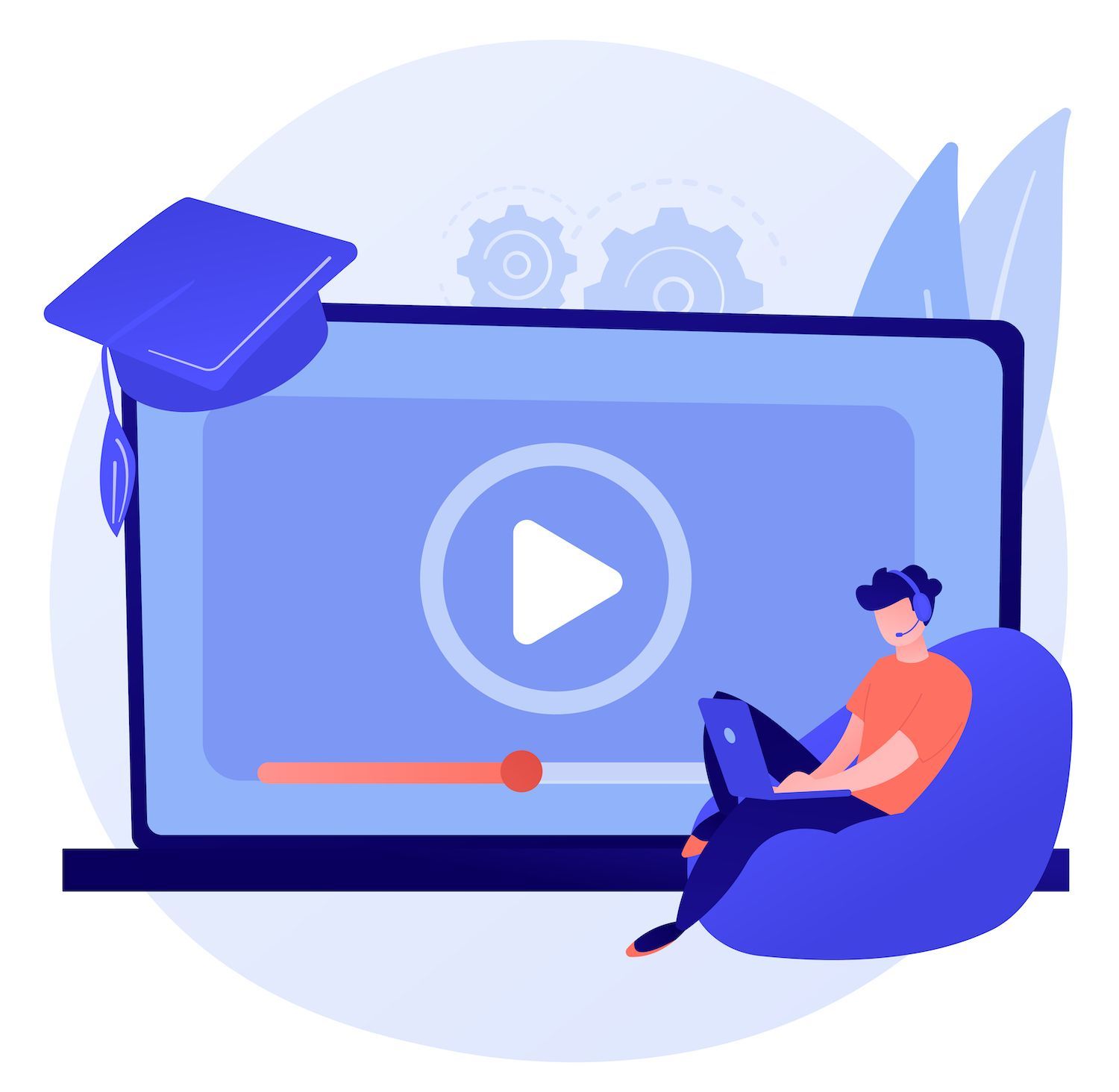
Summary
If a website or application is made by an organisation or a developer on their own, APIs play an important role in the success of their project. However, using them can prove difficult.
- Easy setup and management of the dashboard My dashboard
- Support is available 24/7.
- The most efficient Google Cloud Platform hardware and network powered by Kubernetes to ensure maximal capacity
- An extremely high-end Cloudflare integration to improve speed as well as security
- Reaching a global audience with the possibility of having 35 data centers and 275 PoPs worldwide
This post was posted on here