Mastering React Conditional Rendering Deep Dive (r)
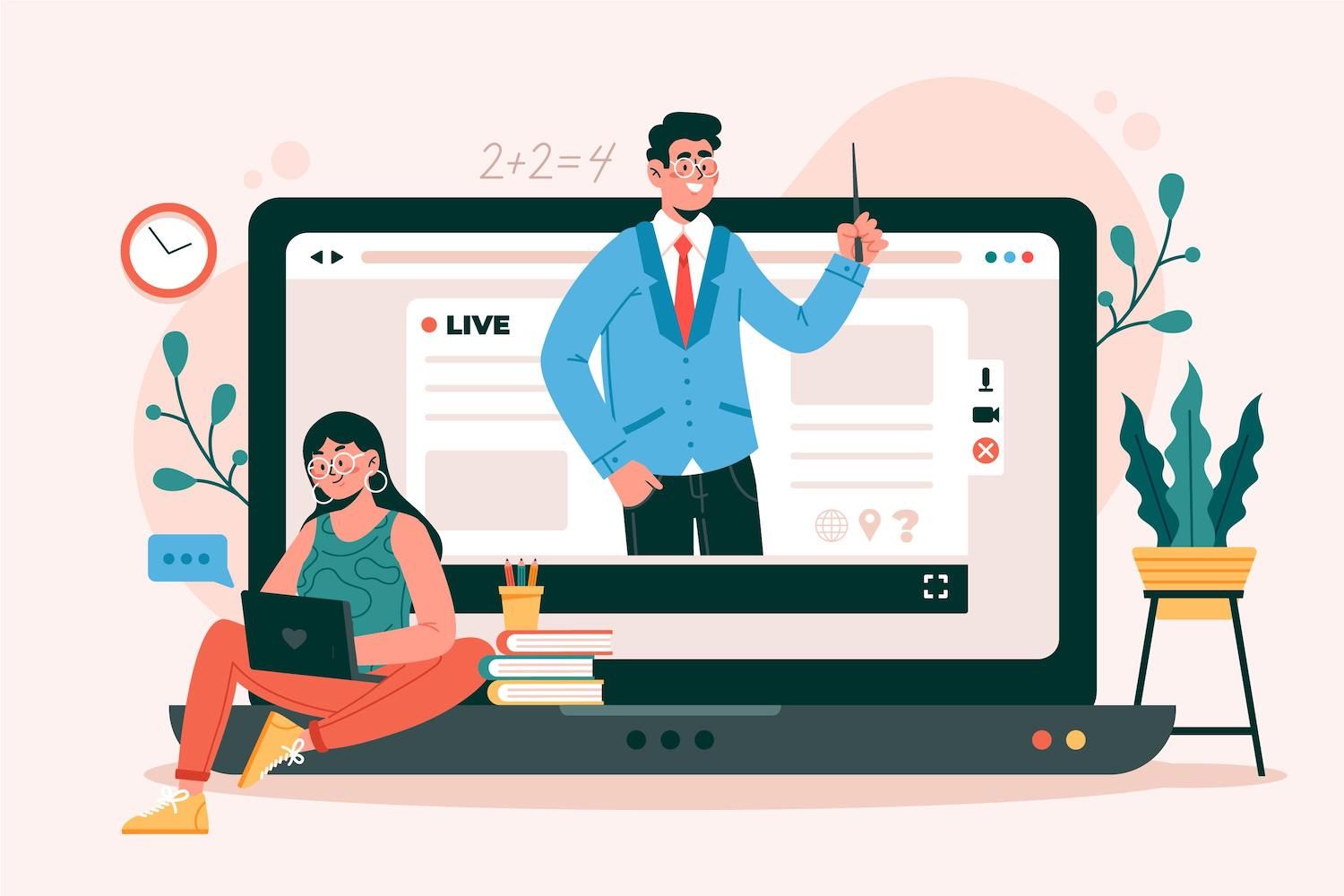
Send this to
Conditional rendering can be described as an extremely vital characteristic of React which allows programmers to render components based on certain situations.
It's an essential notion that is crucial in building websites that are both dynamic and enthralling.
In this complete guide We will plunge into the world of conditional rendering using React and will cover both basics and advanced methods and provide examples to aid in understanding.
React's understanding of Conditional Rendering
It's extremely useful for situations where you wish to disable or block specific UI elements, modify the design of a webpage or present diverse versions of the pages in response to the interaction of the user.
Conditional rendering is essential for React applications since it allows the creation of interactive and dynamic user interfaces that respond to any changes in data or user interaction in real-time.
The Basics of Conditional rendering
There's a myriad of fundamental techniques you can use to create conditional rendering with React. This article will explore each one thoroughly.
Using the if statement to achieve conditional rendering
One of the most simple ways to make use of conditional rendering within React is to make use of the traditional when
statement.
If (condition) return Expression 1; else return Expression 2.
The the if
statement is a good option inside your component's rendering()
method to make sure that the content is rendered in a specific way according to the particulars of the underpinning.
As an example to illustrate, you could use the if statement to show the spinning wheel while you wait for data to load:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const MyComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => // Fetch data from an API fetch('https://example.com/data') .then((response) => response.json()) .then((data) => setData(data); setIsLoading(false); ); , []); if (isLoading) return ; return /* Render the data here */; ; export default MyComponent;
In this case, MyComponent
fetches data from an API making use of the. utilizeEffect
hook. While waiting for the data to be loaded the Spinner component by using the If
statement.
Another example would be rendering as a fallback interface when rendering fails because of an error. your component.
const MyComponent const MyComponent ( data ) * export default MyComponent
In this example it is feasible to make use of MyComponent. MyComponent
that takes in a prop for data
prop. In the event that the prop is not true, the data
prop isn't valid it will display an error message with an "If"
statement.
Additionally, you could display other content to multiple user roles through the when
clause:
const MyComponent = ( user ) * Export default MyComponent;
In this instance, it's feasible to construct a MyComponent
that makes use of a human being for the
prop. Based on the user.role
property, the content is displayed by using the If
clause.
Utilizing the Ternary Operator to Perform Conditional Rendering
The best method to make use of conditional rendering within React is by using the operator the ternary (?) inside JSX.
The ternary operator permits users to compose an inline If-Else by specifying three operands. The first operand is known as the condition. The third and second operands form the expressions. When the condition is true,
then the first expression will be executed. If there is no requirement, the second one will be executed.
For instance, you can render parts of the frame of a prop
import ComponentA from './ComponentA'; import ComponentB from './ComponentB'; const ExampleComponent = ( shouldRenderComponentA ) => return ( shouldRenderComponentA ? : ); ; export default ExampleComponent;
In this code, we have an ExampleComponent
that takes a prop called shouldRenderComponentA
. The ternary operator can be employed to make a conditional rendering of ComponentA
or ComponentB
dependent upon the prop's value.
It is possible to render other texts based on:
import useState from 'react'; const ExampleComponent = () => const [showMessage, setShowMessage] = useState(false); return ( setShowMessage(!showMessage)> showMessage ? show message" message" showMessage ? Hello, world! : null ); ; export default ExampleComponent;
In this instance, it's the ternary operator which renders different text depending upon showMessage's showMessage
status. If the button is clicked, the showMessage value showMessage
may be altered and the text can be displayed or hidden in accordance with the condition.
Additionally, you can make a loading spinner while you download data:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const ExampleComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => const fetchData = async () => const response = await fetch('https://jsonplaceholder.typicode.com/todos/1'); const jsonData = await response.json(); setData(jsonData); setIsLoading(false); ; fetchData(); , []); return ( isLoading ? : data.title ); ; export default ExampleComponent;
In this example it is used to use the ternary operator in order to render a loading spinner while the data is downloading from an API. When the data is downloaded, and rendered, we then render the title of the property
property using the operators that are the ternary.
Employing Logical OR Operators for performing Conditional Rendering
It is also possible to use also the AND ( &&
) as well as OR ( ||
) operators to allow conditional rendering inside React.
A logic AND operator enables the rendering of a component only when one of the requirements is met, while the logic OR operator permits rendering of a part in the event that both or one of the requirements are fulfilled.
They are useful in cases where you need to establish easy rules for determining what elements should be rendered or not. This is for example, if you need to render a button, if the format is acceptable, then you can apply the logic AND operator as is as follows:
import useState from 'react'; const FormComponent = () => const [formValues, setFormValues] = useState( username: "", password: "" ); const isFormValid = formValues.username && formValues.password; const handleSubmit = (event) => event.preventDefault(); // Submit form data ; return ( setFormValues( ...formValues, username: e.target.value ) /> setFormValues( ...formValues, password: e.target.value ) /> isFormValid && Submit ); ; export default FormComponent;
This example is part of the FormComponent
using a form that includes two input fields that allow users to input a username
as well as password
. The form is using useState as the useState
hook to handle the value of the form as well as the the isFormValid
variable, which determines how much each field is filled with value. By using the logic AND operator (&&), we'll render the submit button only when the variable isFormValid
is the case. This will ensure that the button can only be enabled if the confirmation of the forms has been successfully completed.
Similar to that, however you could also try an OR operator to display an error message when data is still loading or a message of error if you are faced with an error
import React, useEffect, useState from 'react'; Const DataComponent = () => const [data setData, data] = useState(null) Const [isLoading isLoading, setIsLoadingisLoading, setIsLoading] = useState(true) setErrorMessage, const, setErrorMessage] = the useState (''); UseEffect(() to fetch data, return to retrieve the data, []); return ( >ErrorMessage ? ( errorMessage ) : ( data.map((item) => ( item.name )) ) > ); ; export default DataComponent;
In this case, DataComponent. DataComponent
collects data through an API by using fetch. It presents the information in an array. It uses an UtilizeState
hook to manage the state of the data, its load state and error message. By using the logic OR operator, (||), we are able to display the loading or error message when one of the situations is fulfilled. A message is shown for the user to indicate what's happening during the process of getting information.
Utilizing the logic AND and OR operators to render conditional content React can be trusted and an easy method of dealing with simple circumstances. However, it is advised to employ other strategies for tackling complex situations, such as shifting
declarations for greater complexity in thinking.
Advanced Techniques for Conditional Rendering
Conditional rendering within React is more complicated according to the specifications of the application. They are sophisticated methods that can be employed to render conditionally in more complex circumstances.
Making use of Switch Statements to render conditional content
Although ternary and statements as well as operators are common approaches to render conditional statements using the switching
statement could be suitable for situations involving multiple situations.
Here's an example
Import React from'react";const MyComponent = (userType) =switch (userType) case "admin": Return Welcome admin user! The case 'user' returned"welcome to regular users!" Return by default. login to learn more. ; ; export default MyComponent;
In this instance, it is the switch
statement is utilized to render the content in line with the userType
prop conditionally. This technique can be useful for a variety of scenarios and offers a more structured and understandable way to manage complex algorithmic processes.
Conditional rendering with React Router
React Router could be described as an application well-known by the general public, which manages routing for clients using React applications. React Router allows you to render the components in accordance with the condition of your current route.
Here's an example of implementing conditional rendering using React Router:
import useState from 'react'; import BrowserRouter as Router, Route, Switch from 'react-router-dom'; import Home from './components/Home'; import Login from './components/Login'; import Dashboard from './components/Dashboard'; import NotFound from './components/NotFound'; const App = () => const [isLoggedIn, setIsLoggedIn] = useState(false); return ( isLoggedIn ? ( ) : ( ) ); ; export default App;
In the code below in the case above, it will utilize the the isLoggedIn
state to render one component, if it's in the Dashboard
component, but only if users are logged in and not it is the NotFound
component when users have not logged into their account. Login component login
component changes its status to show that it's currently in an
condition to change to the situation
when users log into their account.
The child prop of the component to be sent to Login in the form of a Login
component, as well as making use of the settingIsLoggedIn
function. It is possible to add the Login component with props without needing to declare this within the Login
component without specifying the the location of the
prop.
Summary
Conditional rendering is an efficient method in React that lets you alter the user interface based on different conditions.
If you're looking at the degree of sophistication the application's UI algorithm has and you're in the position to choose the algorithm best suited to your requirements.
Be sure to keep your code clean, neat and clean, as well as ensuring that you thoroughly examine your algorithm for conditional rendering to make sure it is working exactly as you would expect it to in various situations.
This post was posted on here
This post was first seen on here