Make the URL shortener which is simple Utilizing Python (r) (r)
URL Shortener
Input an https URL: Enter the created URL"/form>>" Body>/html>
The above code creates an online form that has 2 labels and two input fields of input and one button.
The first input field dubbed URL
is where you type the URL you want to use, in the second field, you'll write the shorter URL.
The address
input field includes the following attributes:
name
To identify the component ( e.g. URL)placeholder
for displaying the URL of an illustration- pattern: To define the pattern of an URL that has the format https ://. *
is necessary
to provide an input URL prior to transmittingvalue
Look at the URL that you previously used
In the second field, there is a value attribute. The attribute is known as value. This attribute is one, which can be utilized to determine the attribute new_url.. New_url is an uncomplicated URL.
is an uncomplicated URL generated through the shorteners within the pyshorteners library, which is found within the main.py file (shown in the section below).
This entry form is identified by the following image:
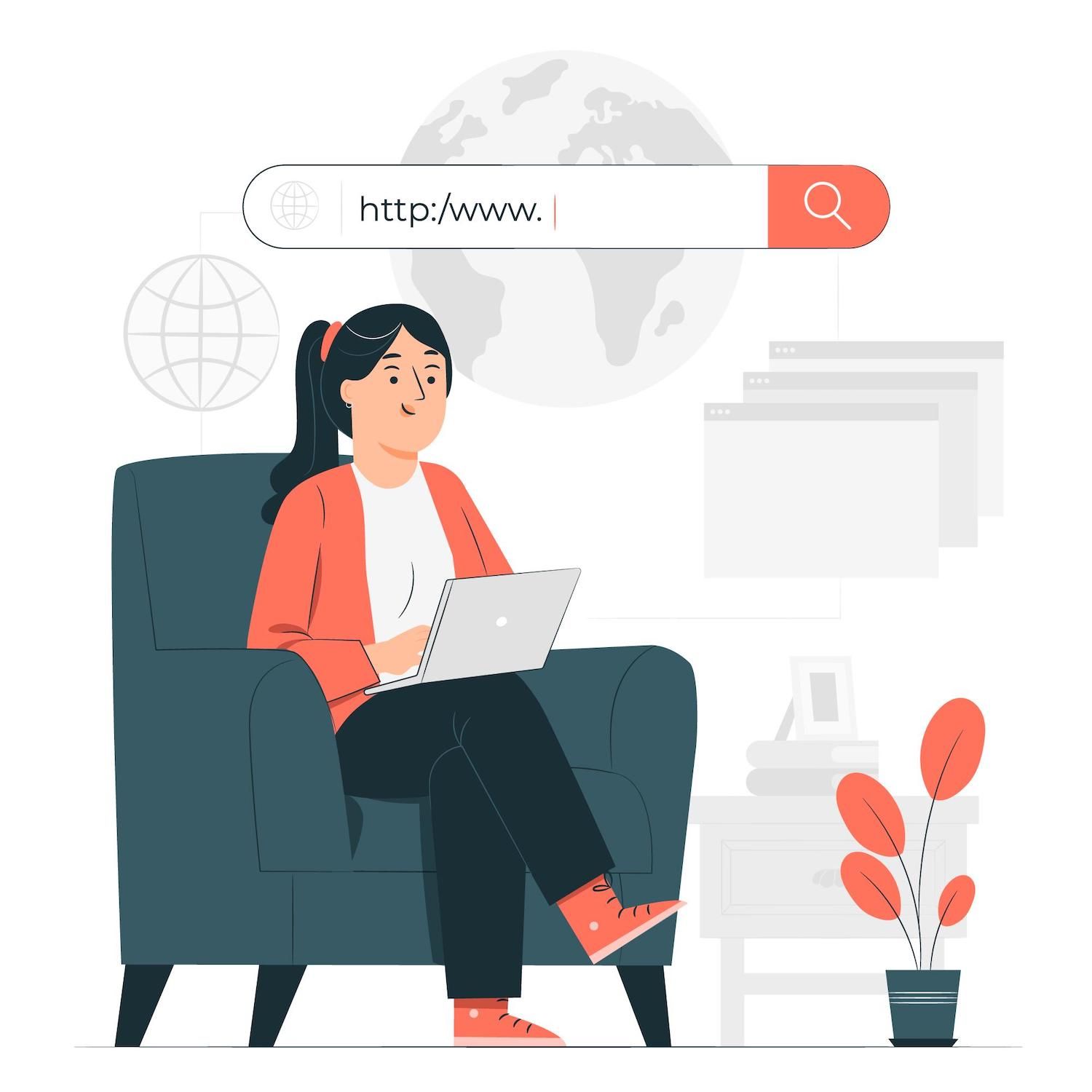
URL Shortening Code Using pyshorteners
When you've completed the form, you'll be able to modify the form more useful with Python and shortereners with Python.
This code transforms the lengthy URL into smaller URLs and run the web-based application. Go to the main.py file you made earlier. Then, include the code in the below section to save it. Save it to:
from flask import Flask, render_template, request import pyshorteners app = Flask(__name__) @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] short_url = pyshorteners.Shortener().tinyurl.short(url_received) return render_template("form.html", new_url=short_url, old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
The code above will load the shorteners in the to be found in the pyshorteners library as well as the following modules that are part of the Flask framework. They are required to reduce URLs:
Flask
Flask Flask framework has been previously released.- render_template HTML0 render template HTML0 render template is a rendering program which generates HTML documents. HTML documents are generated by using directory templates.
template
. request
A class inside the Flask framework, which stores all the data that the user transmits through the front-end of the app to the backend of its application, for example an HTTP request.
Then, it creates an application known as home()
that takes URLs that are entered in the form, and generates the abbreviated URL. It is possible to use the app.route()
decorator could be employed in order to associate the function with the specific URL location to execute the app. The POST/GET methods deal with the requests.
Within"home ()", the "home()
function it contains an in between
and an expression that is conditional.
For the if
statement, if request.method=="POST"
, a variable called url_received
is set to request.form["url"]
, which is the URL submitted in the form. This is due to the fact that URL
is the name that is assigned to the input field that is specified within the HTML form, which was developed in the past.
Then, a variable called short_url
is set to pyshorteners.Shortener().tinyurl.short(url_received)
. Two methods use the shorteners in the pyshorteners library: .Shortener()
and .short()
. The .Shortener()
function is used to create a instance of the pyshorteners class instance. Additionally, its .short()
function is used to input the URL for an input, and also to reduce the URL.
Short() function, also known as the short()
function, tinyurl.short()
is among the shorteners which are a integral to the shorteners in the shorteners in the pyshorteners libraries. These have many APIs. osdb.short()
is another API that could be utilized to accomplish similar purposes.
Its render_template()
function is employed to generate the HTML template form.html file. form.html and send URLs back to form with arguments. The function makes use of arguments. "new_url"
argument has been changed to the short_url argument
as well the argument for old_url
is added to the URL you are receiving
. The declaration's definition has been extended.
declaration's scope is extended to.
If you make use of the alternative
statement to find out whether the method used to request differs from POST the request will display using form.html HTML template. form.html HTML template will be shown.
An example of URL Shortener Web Application built by using Python Shorteners Library. Python Shorteners Library
To demonstrate the pyshorteners URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
Enter a hyperlink you like to the right on the form for web:
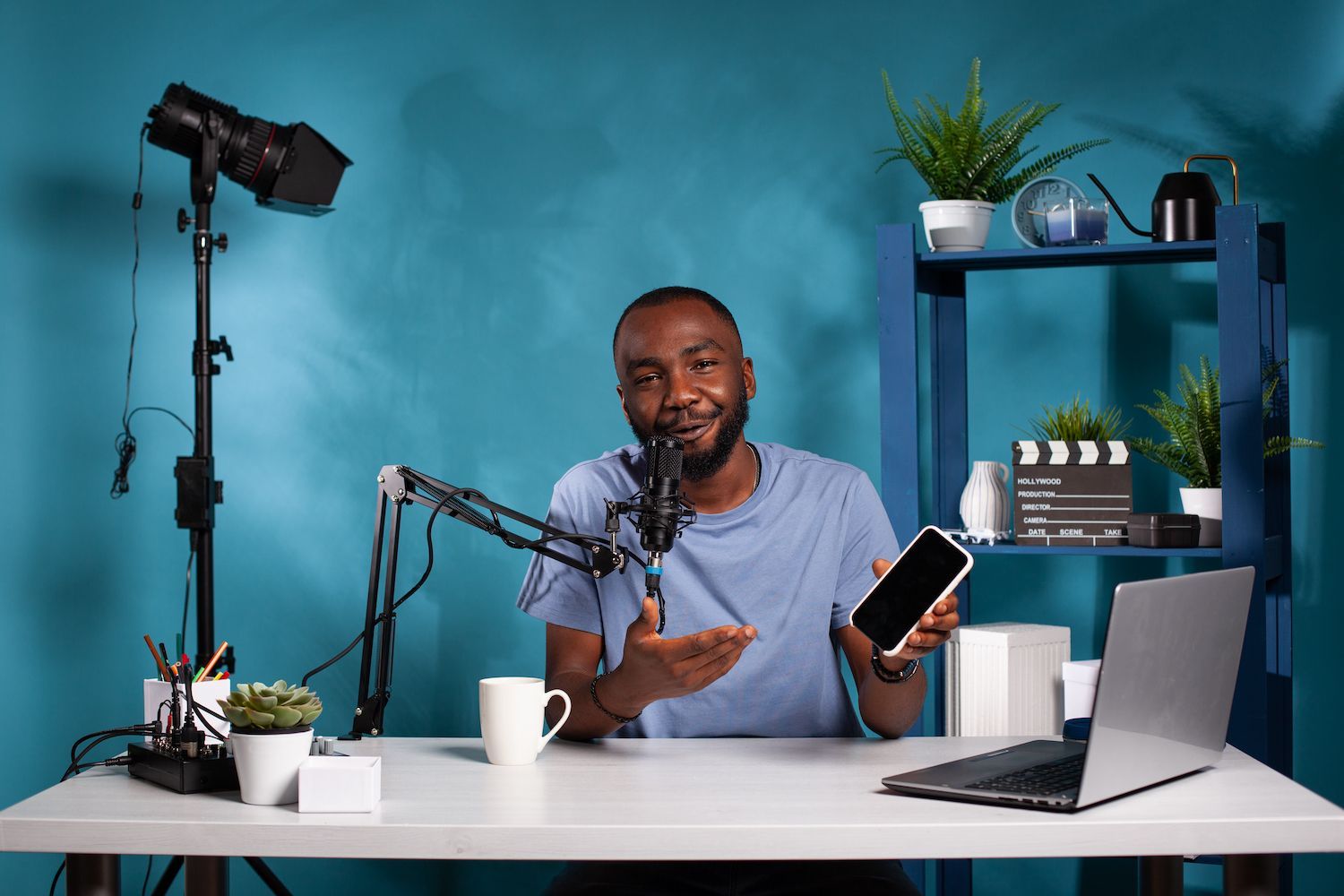
Hit on the link to send the button to create URL. Click Submit to create the URL as a version that is smaller in size. Use tinyurl
as the domain name in the box that states the URL that was created.
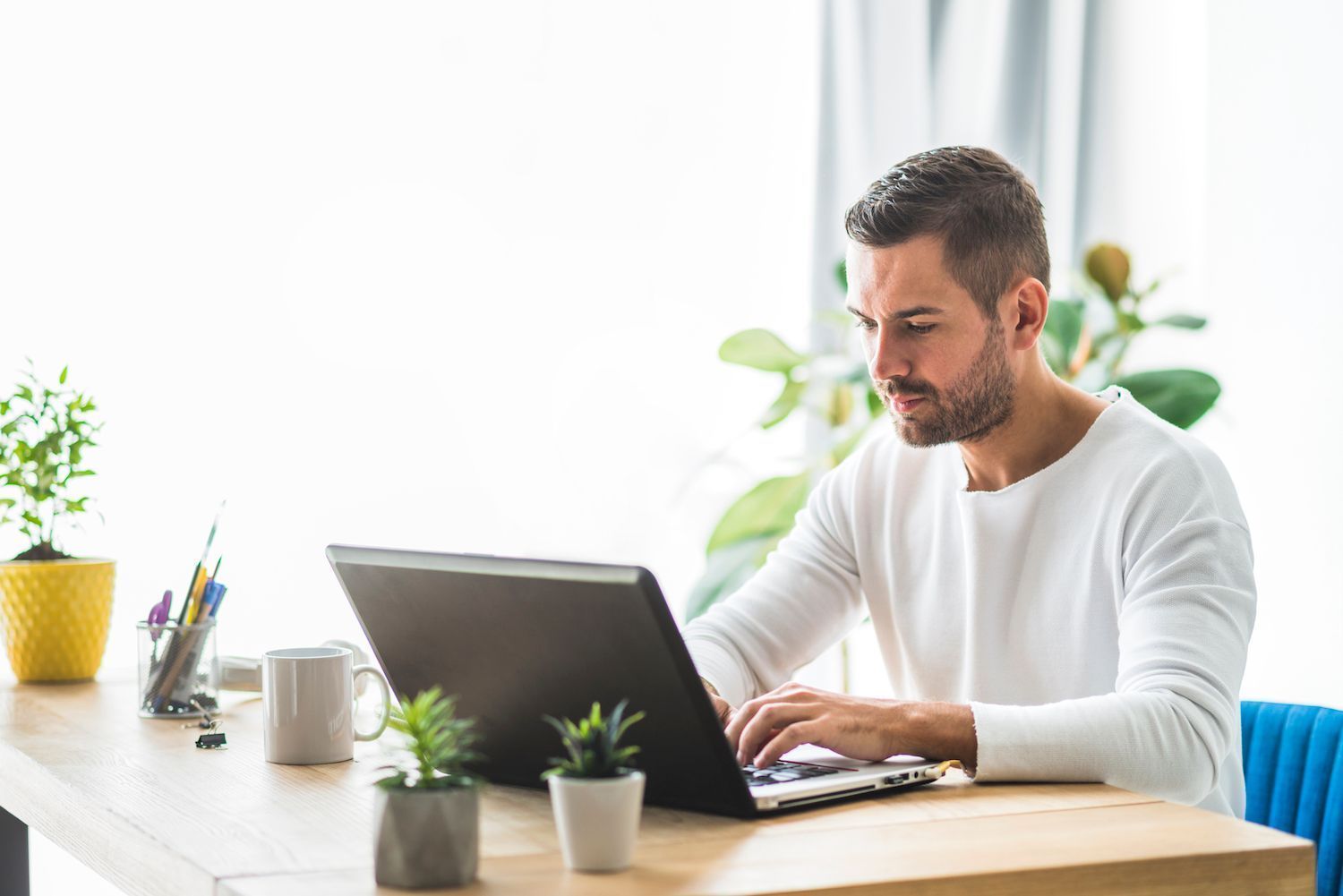
Utilizing Bitly API Module Bitly API Module is now in a position to create this URL Shortener App for use to shorten URLs on the Web. App
In this class, you'll develop a URL-shortening application with the help of Bitly API. Bitly API. Bitly API. Bitly API module provides an alternative method to shorten URLs. It also provides detailed information about clicks, the location of users along with the type of device that is being used (such such as a mobile device or desktop).
Set up Bitly API. Bitly API. Download and install the Bitly API using the following steps:
pip to install Bitly API-py3
Access tokens are needed to access to the Bitly API. They are access tokens that are accessible when you sign into Bitly..
After you've completed the registration procedure, you can sign up for Bitly. Once you've completed your sign-up process, login to Bitly to access your account dashboard.
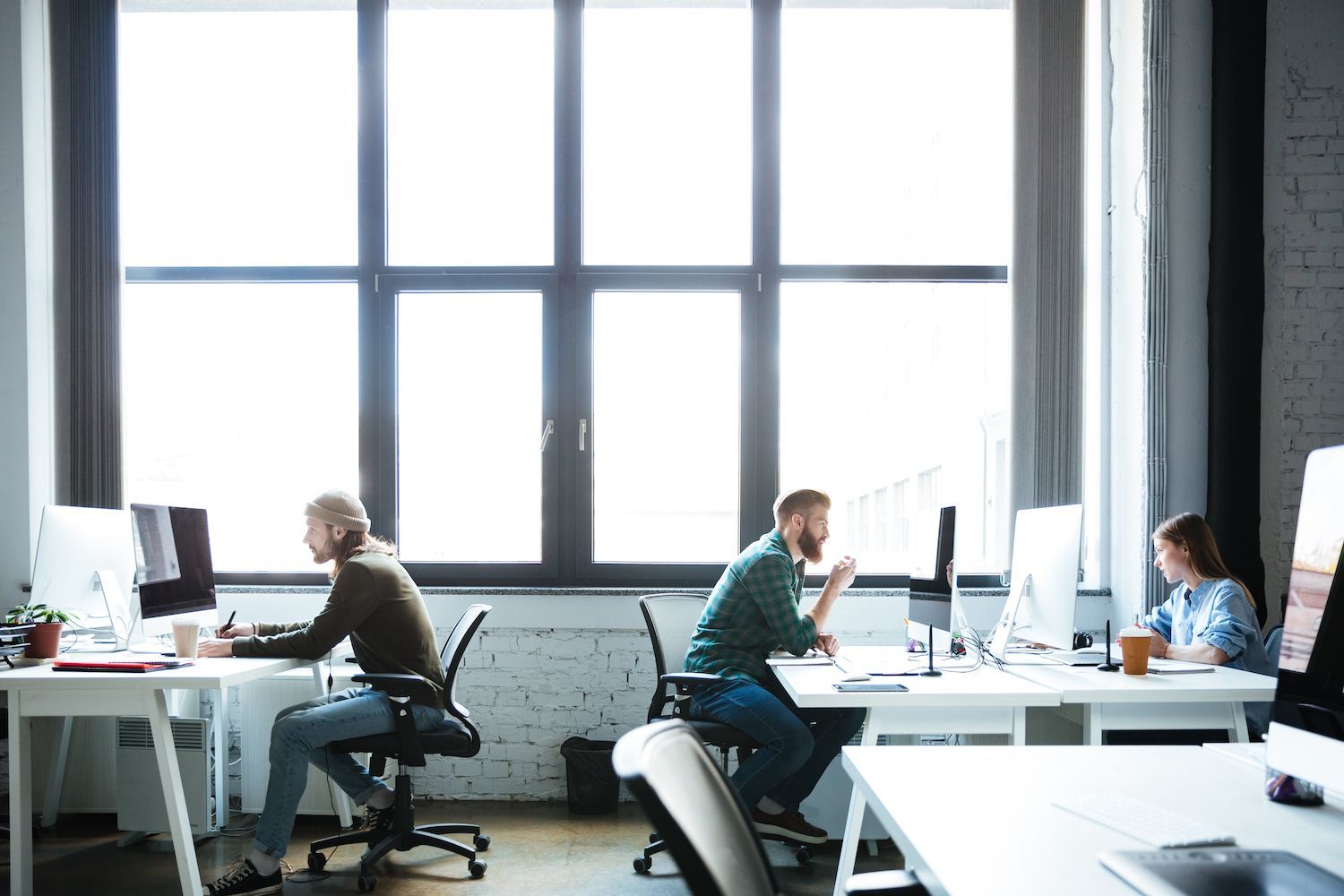
Choose Settings in the sidebar to the left. Then, you can browse sections of the API section located within Developer settings.
Create an access token through entering the username in the space just above the generator token button. It is evident on the following image. Keep the token in mind for later use in your application:
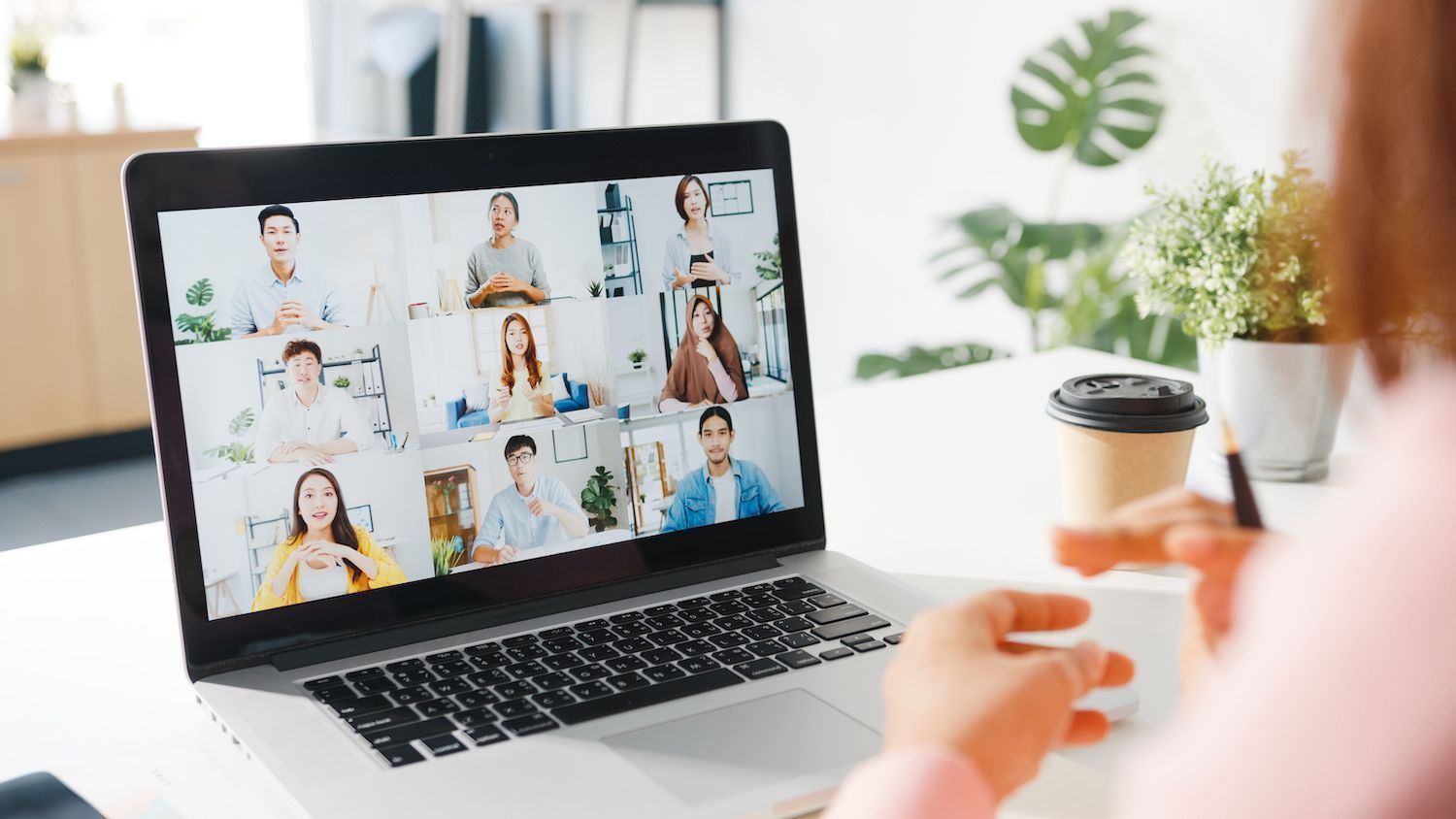
URL Shortening Code Using the Bitly API
Once the Bitly token has been received Bitly token via Bitly You'll then be able to create a program for your site application to make the URL shorter via Bitly API. Bitly API. Bitly API.
The same template you created to build the shortereners section, with a few changes made to your main.py file:
from flask import Flask, render_template, request import bitly_api app = Flask(__name__) bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxxxx" @app.route("/", methods=['POST', 'GET']) def home(): if request.method=="POST": url_received = request.form["url"] bitly = bitly_api.Connection(access_token=bitly_access_token) short_url = bitly.shorten(url_received) return render_template("form.html", new_url=short_url.get('url'), old_url=url_received) else: return render_template('form.html') if __name__ == "__main__": app.run()
In the case above, bitly_api
is loaded using import bitly_api
. The access token is then saved in a variable called bity_access_token
, as in bitly_access_token = "37b1xxxxxxxxxxxxxxxxxxxxxxxx"
.
"home"() function is a shortening of the URL. "home"()
function is a contraction of URL. Additionally, it includes the "in-case-of-nothing"
conditional expression.
When you are using this statement, you must use the
statement when POST is your preferred method of communication or the request has the format of POST
The URL you enter on the form will change to correspond with requested URL
variable.
The bitly_api.Connection(access_token=bitly_access_token)
function connects to the Bitly API and passes it the access token you saved earlier as an argument.
In order to reduce the length of URLs it is possible to make use of the bitly.shorten() function. bitly.shorten()
function. It is a method to reduce the length of a website by supplying the the URL_received
value as an argument, and then storing the results in an array known as the short_url
.
The form is then rendered and then URLs return for display in the form. The rendering process is made by making use by the rendering template()
function. Once you have submitted the form, this when
phrase is the primary part on the application form.
If you use the the other
clause of the assertion, the model will render using rendering_template() function. render_template()
function.
Examples for URL Shortener Web Application built by Bitly API. Bitly API
To demonstrate the Bitly API URL shortener application, navigate to the default route for the application, http://127.0.0.1:5000/, after running the application.
You can input a favorite link within the box at the top of the form for websites:
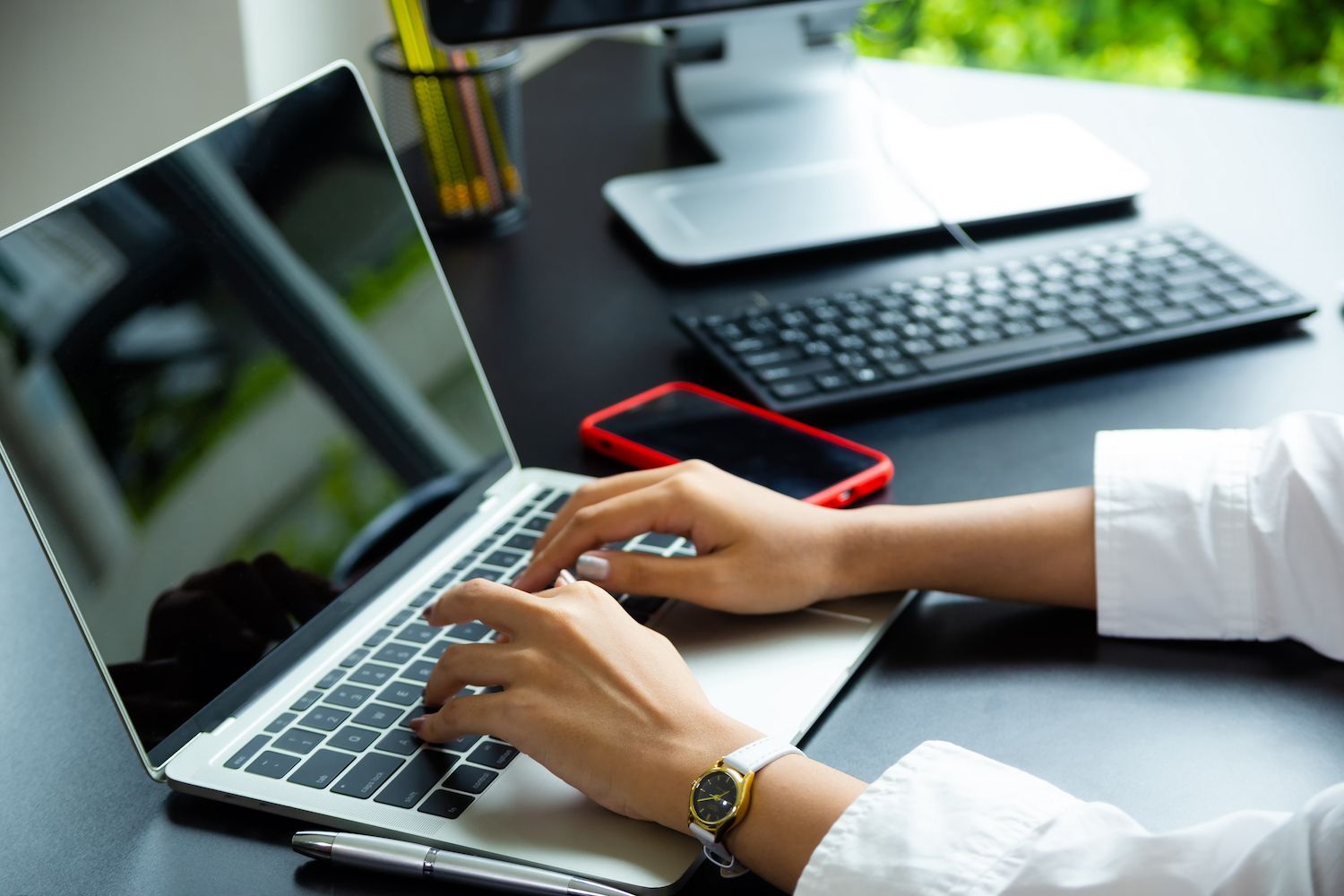
Click "Submit" to generate a URL that uses bit.ly
as the domain. The second part is the website application
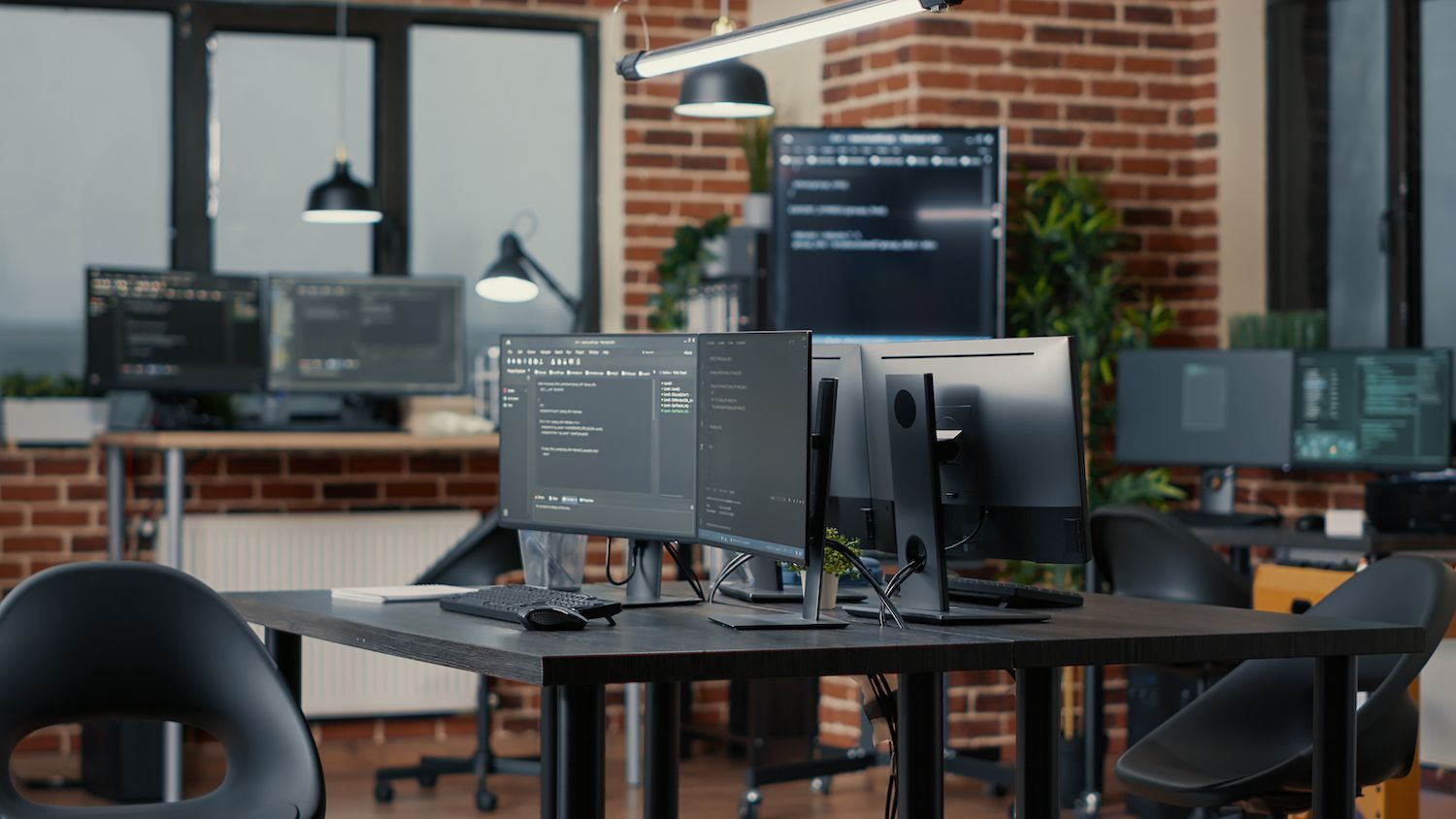
Utilizing Bitly API to use the Bitly API to use the Bitly API to shorten URLs in your Python program is as easy to do as it sounds.
Summary
URL shorteners provide you with short URLs that users are able to use and are more attractive and take up less space. In this article you'll discover more about URL shorteners, as well as their advantages as well as ways to build an URL shortener-related website application using Python via Pyshorteners and the Bitly API. The Python shorteners library allows for shorter URLs. Bitly API provides detailed analytics. Bitly API provides precise analytics and smaller URLs.
Adarsh Chimnani
Adarsh is a web-designer (MERN stack) He's a fan of gaming-related design (Unity3D) and is an avid lover of anime. He is awed by the knowledge he acquires by curiosity, using the knowledge he's acquired in the workplace and disseminating his knowledge to others.
The post first appeared here. this site
The article was published on this site
This post was first seen on here