How do you debug Node.js Coding Using Multiple Tools
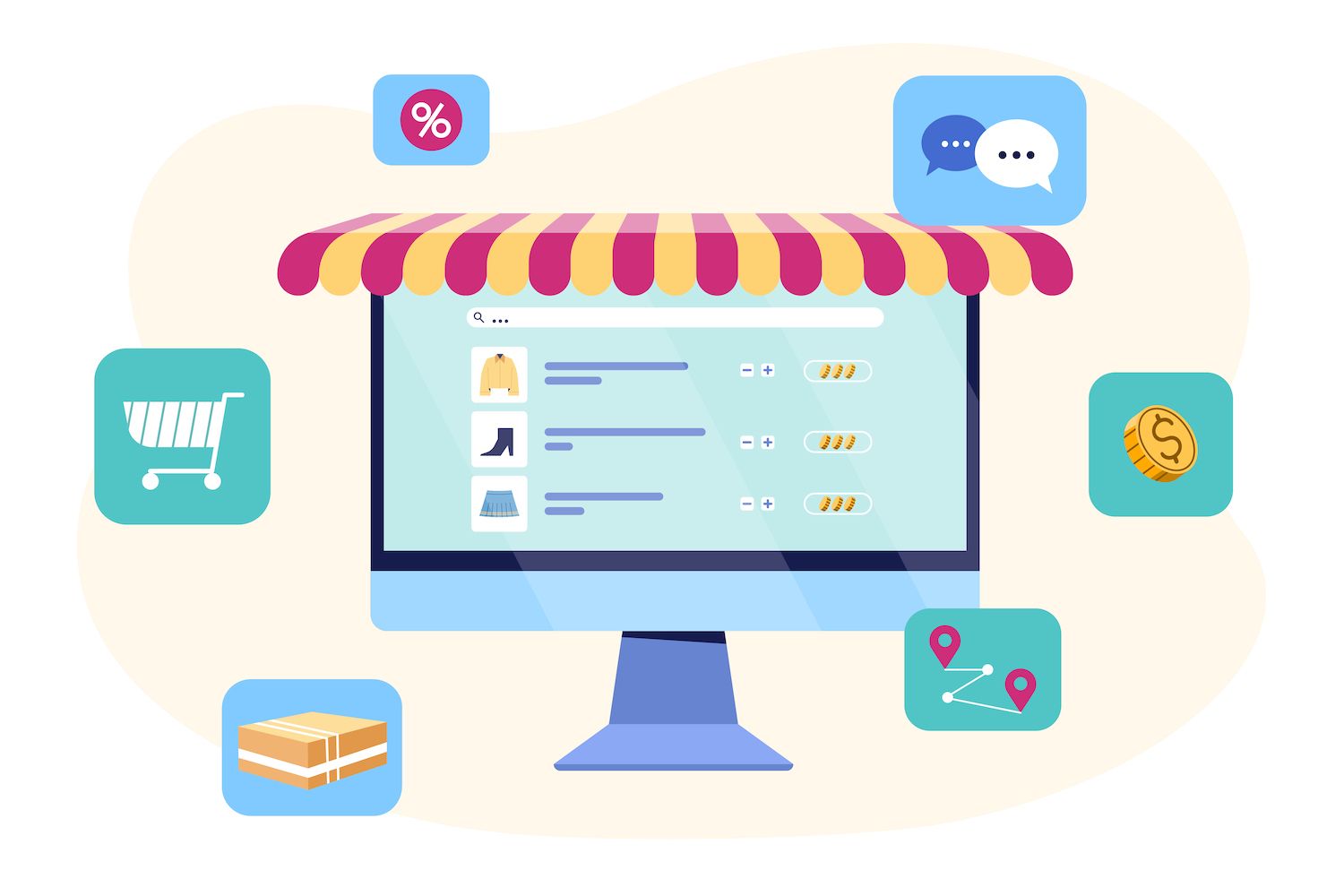
But, developing software can be a difficult task as well as the Node.js code will fail at some point. This tutorial demonstrates various tools to help detect software problems and identify the root of the issue.
Let's begin.
Debugging Overview
"Debugging" refers to the term used to describe the different techniques used to correct software bugs. This process of fixing a problem is usually easy. The root of the issue may be more complex and will require lots of head scratching.
In the subsequent paragraphs, we will discuss three general types of error you'll encounter.
Syntax Errors
The code you create doesn't follow the rules of the language, like when you take out the closing bracket or you mispelling a sentence such as console.lag(x)
.
A good code editor can help spot common problems by:
- False or valid statements are color coded
- Type-checking variables
- Functions for auto-completing and variables with names.
- The brackets need to be highlighted in order to correspond with.
- Auto-indenting code blocks
- Identification of code that is not reachable
- Refactoring complicated tasks
A code linter similar to ESLint will also report syntax mistakes, incorrect indentation, and non-declared variables. ESLint is the Node.js software that you can download across the world using:
npm i eslint -g
It is possible to check JavaScript documents from the command line with:
Eslint mycode.js
...but it's much easier to make use of the editor plugin , such as ESLint to Visual Studio Code and the linter-eslint plug-in for Atom which will instantly validate code as you type it:
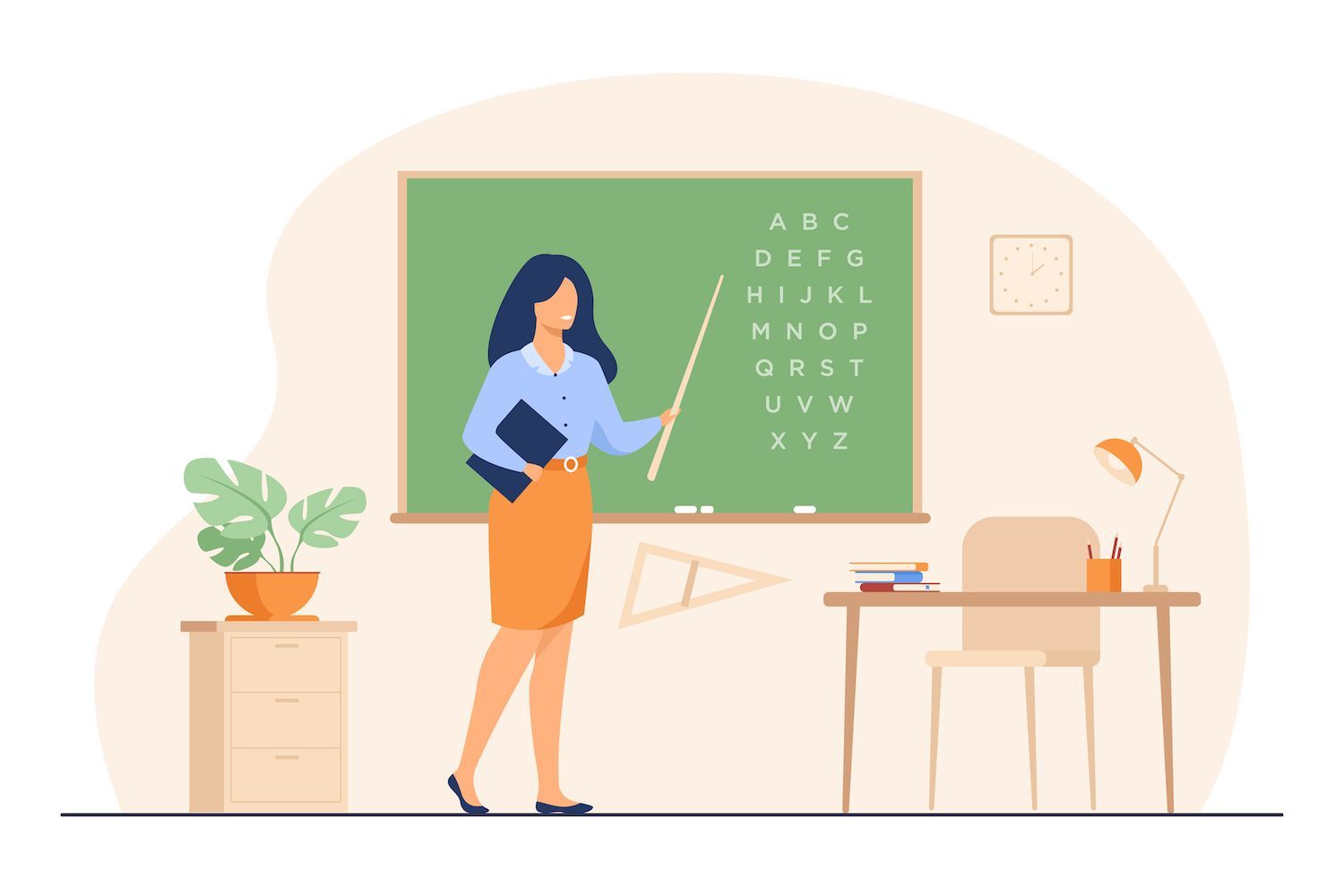
Logic Errors
The program runs, however it's not working the way you'd expect. In this case, for example, the user's login doesn't get completed after they want it to be; the report is showing incorrect numbers the data hasn't been completely saved to a database; and so on.
The reason for logic errors could result from:
- Incorrectly using the variable
- Untrue conditions, e.g.
if (a more than 5)
rather thanthe case of (a greater than 5)
- Calculations which do not include the precedence of an operator e.g.
1+2*3
will result in seven rather than nine.
Runtime (or Execution) Errors
The error is only apparent when the program has been run which can cause the program to crash. Errors during runtime can be caused by:
- The variable to divide by is set to zero
- In the process of accessing an array item that does not exist
- Try to write into a database that is read-only
Runtime and logic errors are harder to identify however, the following strategies for development may help:
- Make use of Test-Driven development: TTD advises you to create tests prior to the moment a function is created, e.g. FunctionY will return X when Z is set for the parameters. Tests are performed in the beginning of development, as well as afterward to confirm that it continues to run as expected.
- Make use of an issue-tracking software:There is nothing worse than an email claiming "Your software does not function"! Issue tracking systems enable you to keep track of certain issues, track reproduction steps, determine prioritization, assign developers and keep track of the evolution of solutions.
There is a possibility of encountering Node.js bugs, but this section will provide methods to spot the elusive issue.
Set Appropriate Node.js Environment Variables
Environment variables defined in the operating system of the host can impact Node.js modules and applications. It is typically the NODE_ENV
that is typically changed to development during debugging or in production mode in the case of being run in the server that is live. Create environment variables in macOS or Linux with:
NODE_ENV=development
or at or at or at (classic) Windows command prompt:
set NODE_ENV=development
or Windows Powershell:
$env:NODE_ENV="development"
The popular Express.js framework setting NODE_ENV to development blocks template cache and also outputs errors in verbose form, which can be helpful when trying to debug. Certain modules may offer similar features as well, so it is possible to add a NODE_ENV option to your application, e.g.
// running in development mode? const devMode = (process.env.NODE_ENV !== 'production'); if (devMode) console.log('application is running in development mode');
Additionally, you can make use of util.debuglog as well. util.debuglog method to produce error messages on a specified base, e.g.
import debuglog from the 'util' const myappDebug = debuglog('myapp'); myappDebug('log something');
The program will only print the log messages when NODE_DEBUG is set to myapp or a wildcard , like * or my*.
Make use of Node.js Line Command Line Options
Node scripts are generally executed via node. This is then followed by the title of the entry script.
Node app.js
You can also define command line options to control the various elements of the running time. Useful flags for debugging include:
--check
Verify the syntax of the script before using the script--trace-warnings
produce a stack trace, which occurs in the event that JavaScript Promises don't solve or reject--enable-source-maps
map source sources using an application that transpilers for example TypeScript--throw-deprecation
warn when warnings are issued when Node.js features are utilized--redirect-warnings=file
Output warnings to a file instead of warnings to a file Not--trace-exit
produce a trace of the stack afterprocess.exit()
is invoked.
Send messages into the Console
Outputting a console message is one of the most straightforward methods to check a Node.js application.
console.log(`someVariable: $ someVariable `);
Some developers are aware that there is a wide range of different console choices:
Console Method | Description |
---|---|
|
standard console message |
|
output object is the result from a short JSON string |
|
the property of objects which print pretty |
|
output arrays and objects in tabular format |
|
an error message |
|
the counter's increment and output |
|
Set a specific counter |
|
make an indentation on an email message |
|
Interrupting a whole group |
|
Starts a designated timer |
|
reports the elapsed time |
|
Stops a timer that is known as |
|
produce an output trace (a listing of all functions named) |
|
Clear the console |
console.log()
can also be used to accept a list of comma-separated values:
let x = 123; console.log('x:', x); // x: 123
...although ES6 destructuring offers similar effects with less effort
console.log( x ); // x: 123
Console.dir() can be described as a command that prints the properties of objects. console.dir() command prints object's properties like util.inspect():
console.dir(myObject, depth: null, color: true );
Console Controversy
Some developers recommend that you shouldn't not utilize console.log()
because of:
- You can alter things, or you may overlook the removal of it and
- There's no reason to do it when there are better alternatives for debugging.
Don't be fooled by anyone who says that they don't use console.log()
! Logging can be a hassle and time-consuming process. Yet, every person uses it at least once during their life. Make use of the technique or tool you would prefer. Fixing a bug is more important than the technique that you use to identify the issue.
Utilize a third-party logging system
Third-party log systems provide advanced features like messages levels, volume, sorting, file output and profiling, as well with reporting, among others. The most popular options are loglevel, pino, cabin, morgan Signale, the storyboard, tracer, and winston.
Utilize the V8 Inspector
The V8 JavaScript engine provides the Debugging program that you can use to test your application in Node.js. Launch an application with node inspect, e.g.
node check app.js
The debugger stops the debugging process when it reaches the end of line. The debug> prompt
$ node inspect .\mycode.js One const number = 10. 3. (i = 0, (i
For help, click Help to see a command list. It is possible to move within the program using:
- or C: continue execution ou C for C: continue execution
- Next or next to run the next command
- step or step step or step function described as
- out or o Move away from the function, and then return to the call statement
- pause: pause running code
- watch('myvar') Watch the variable
- setBreakPoint() or sb(): set a breakpoint
- restart Restart the script
- .exit or Ctrl Cmd Exit the debugger
It's true that debugging is time-consuming and unwieldy. Use it only when there's an alternative, for instance, when you're working on code that's running on an external server, and are unable to connect elsewhere or install additional software.
Use the Chrome Browser for debugging Node.js Code
Do you want to know how we did to increase our volume by more than 1000 percent?
Join the 20,000+ who get our weekly newsletter that contains insider WordPress tips!
If you want to debug a typical web-based application, you must start it by using the option -inspect to activate for the V8 Debugger's Web Socket server:
Node Inspect index.js
Note:
- index.js may represent the entry script for the application.
- Make sure you select
the option to inspect
with double dashes in order to ensure that you do not launch the client for debugging by using text. - You can use nodemon in place of node if you'd like to restart your application when a file is modified.
The debugger can only accept connections from the local device. If your application is running using a different device, virtual machine, as well as a Docker container you may utilize:
node --inspect=0.0.0.0:9229 index.js
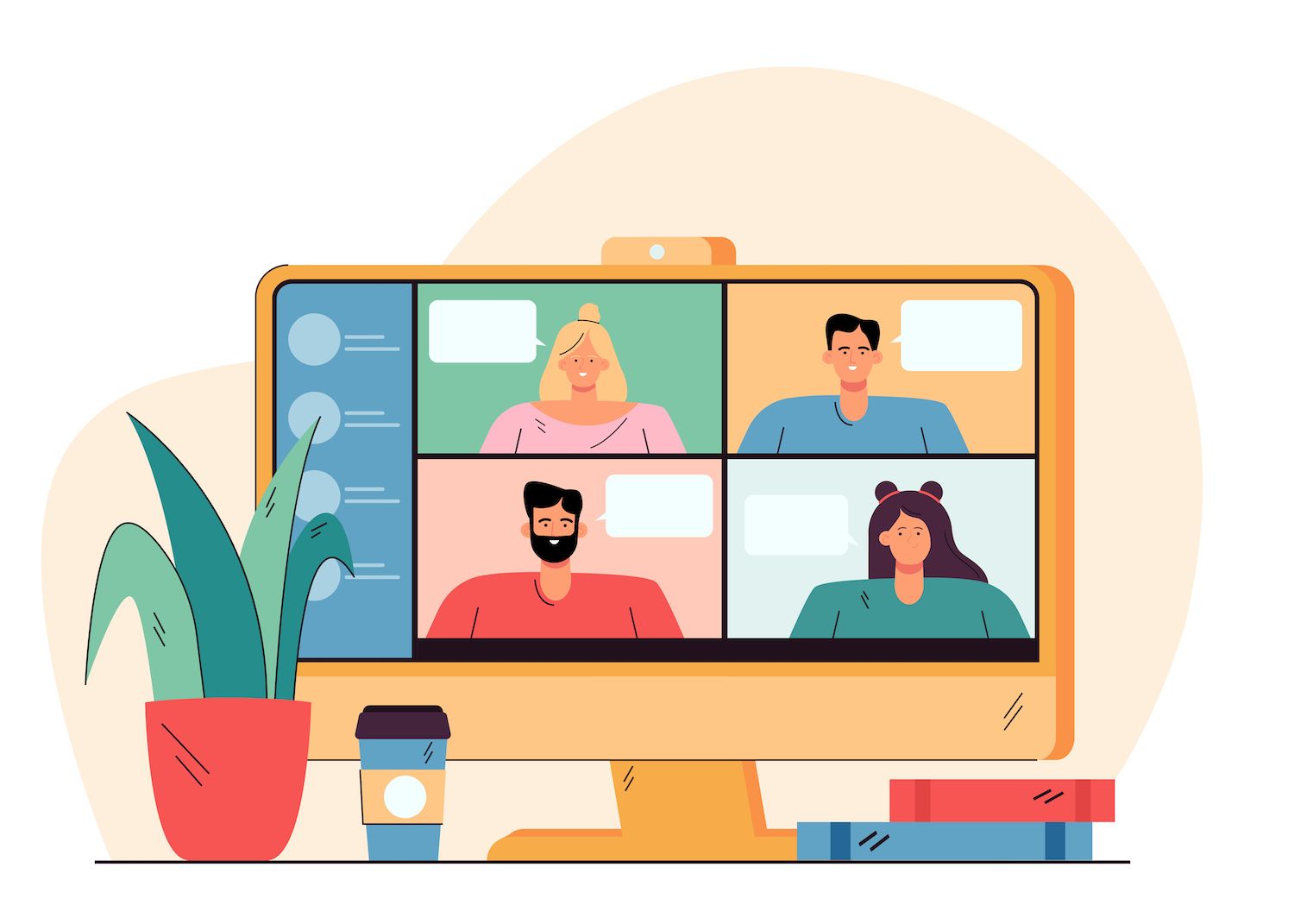
There is also the option to utilize the option --inspect-brk
in place of inspect
to halt running (set the breakpoint) in the initial line, allowing you to step through the code starting from beginning.
Open a browser using Chrome and then type Chrome://inspect
in the address bar to show local and networked devices:
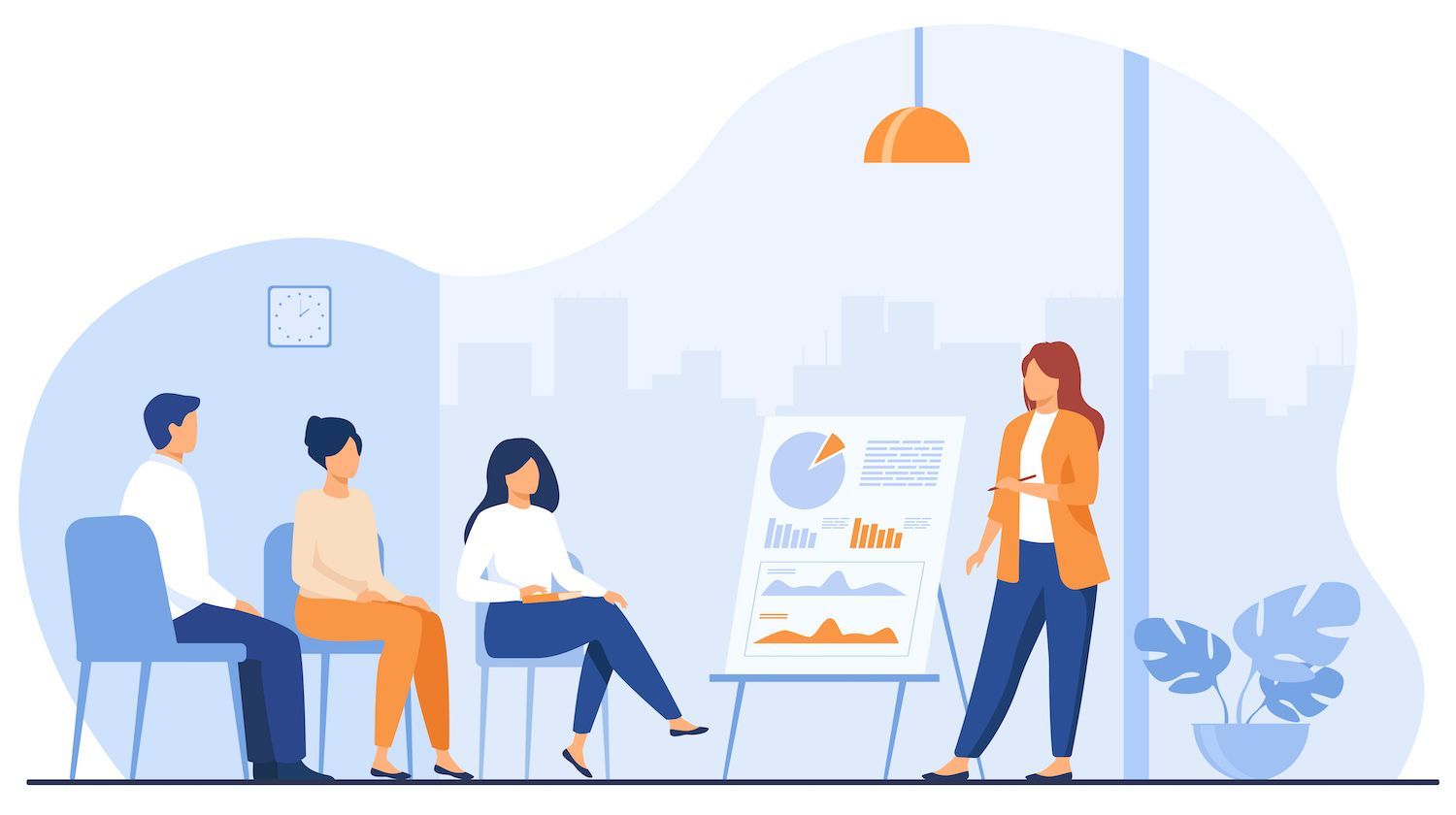
If your Node.js application doesn't display as a Remote Source or you're not able to find it:
- Select Open the dedicated DevTools to Node and select the port and address or
- Look for the network destination that you wish to find. Click to configure add the IP address as well as the port number for the device that it's running.
Use the target's inspect link to launch DevTools' DevTools Debugger Client. It should be well-known to anyone who's utilized DevTools to debug client side issues:
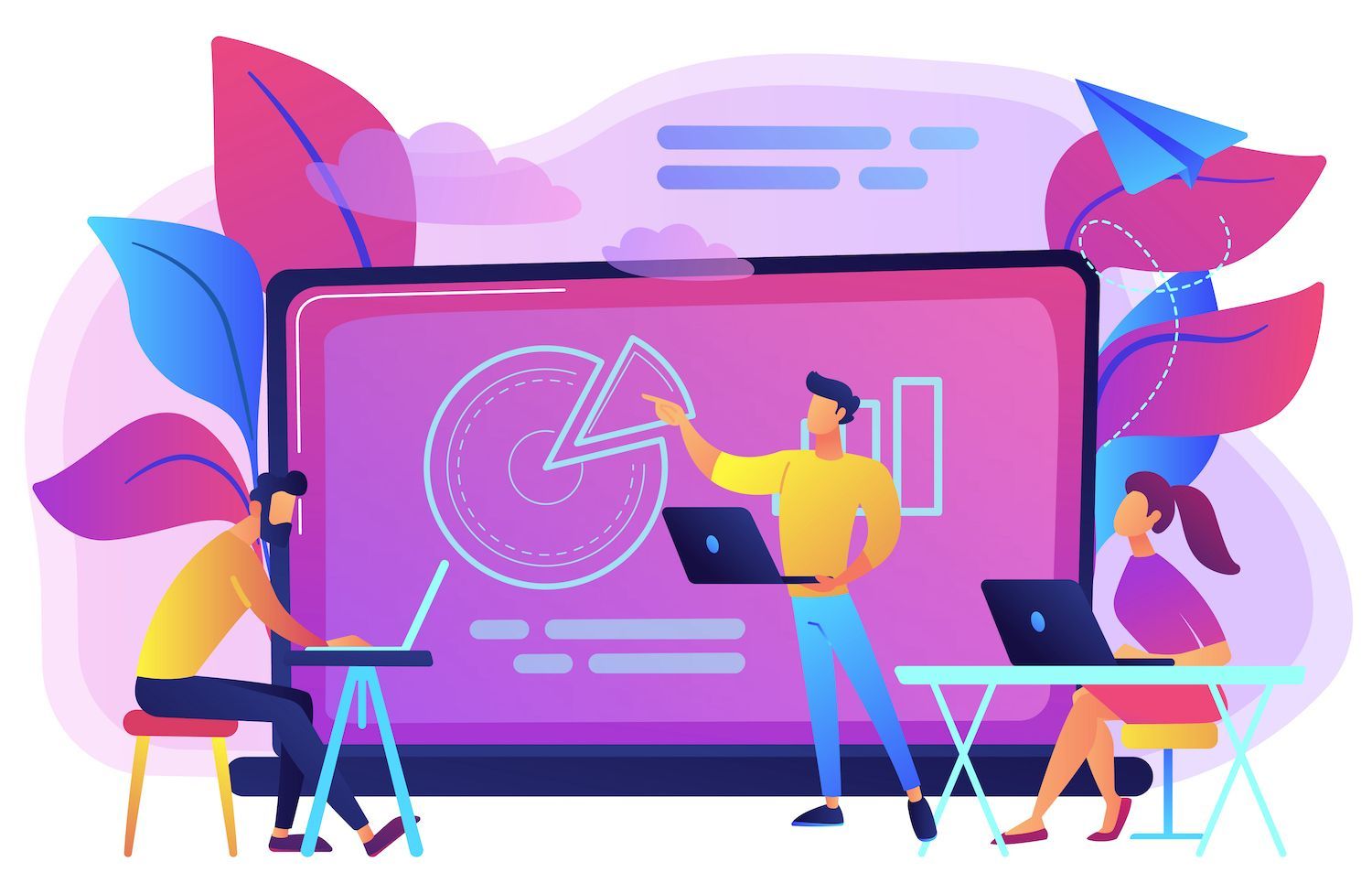
Change on your Sources panel. It is possible to open any file by pressing Cmd Ctrl + P, and after that entering the file's name (such such as index.js).
It's also simpler to connect your project's folder into the workspace. This lets you load files, modify and save your files right via DevTools (whether you think that's an ideal idea is another question!)
- Click + Add folder to the workspace
- Choose the best address to host your Node.js project.
- Click Acceptto allow file modifications to be completed
You can now load files from the left-hand directory tree:
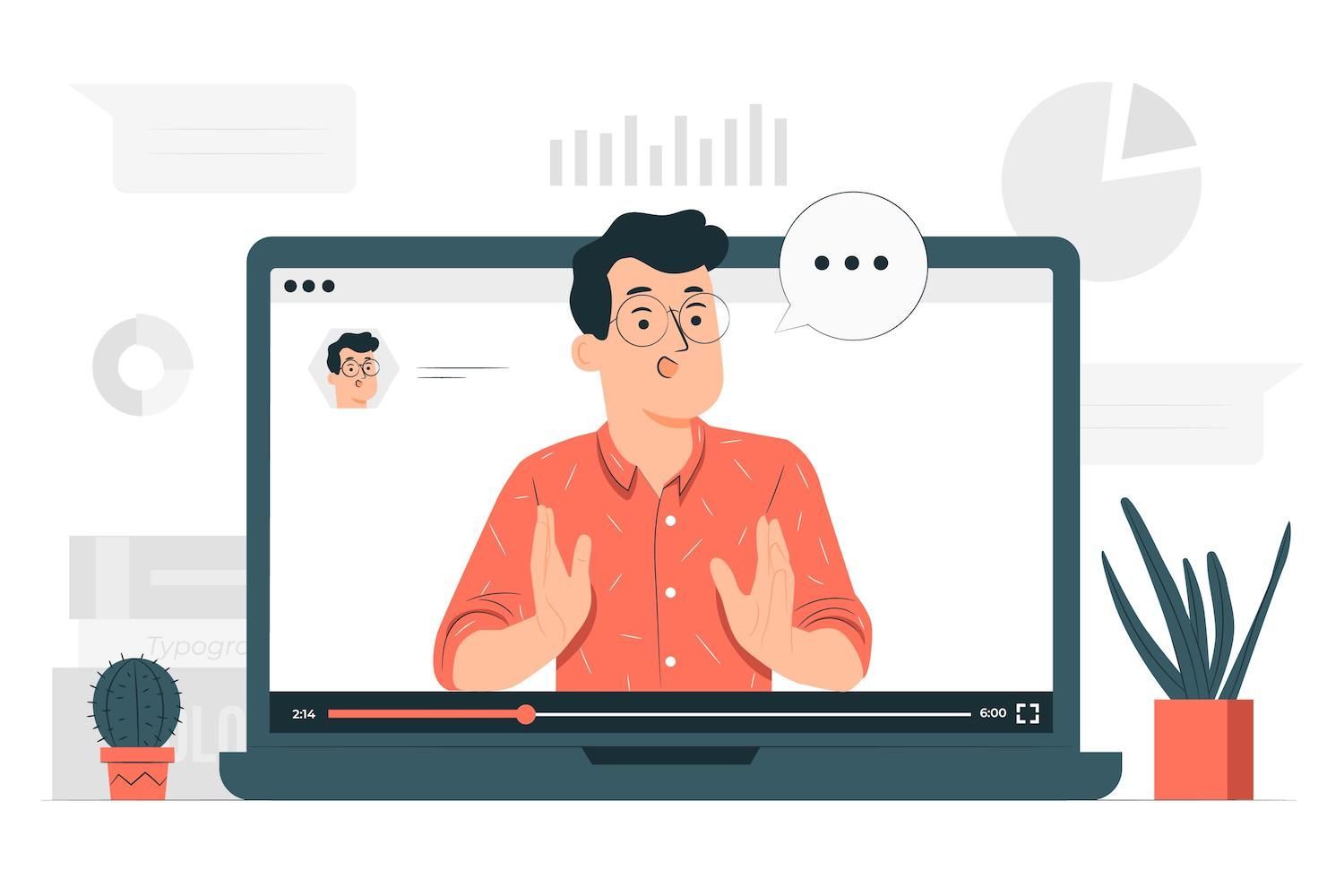
Simply click any line to establish a breakpoint indicated by the blue marker.
Debugging depends on breaks. Breakpoints determine when the debugger is supposed to stop program execution. They also indicate what is happening to the programmer (variables and call stacks, and so on.)
You can define the number of breakpoints you want to define in your user's user interface. Another possibility is to include a debugger; statement into the code that stops once the debugger is attached.
Utilize your web browser to get to the line that a breakpoint was established. In the example here, http://localhost:3000/ is opened in any browser, and DevTools will halt execution on line 44:
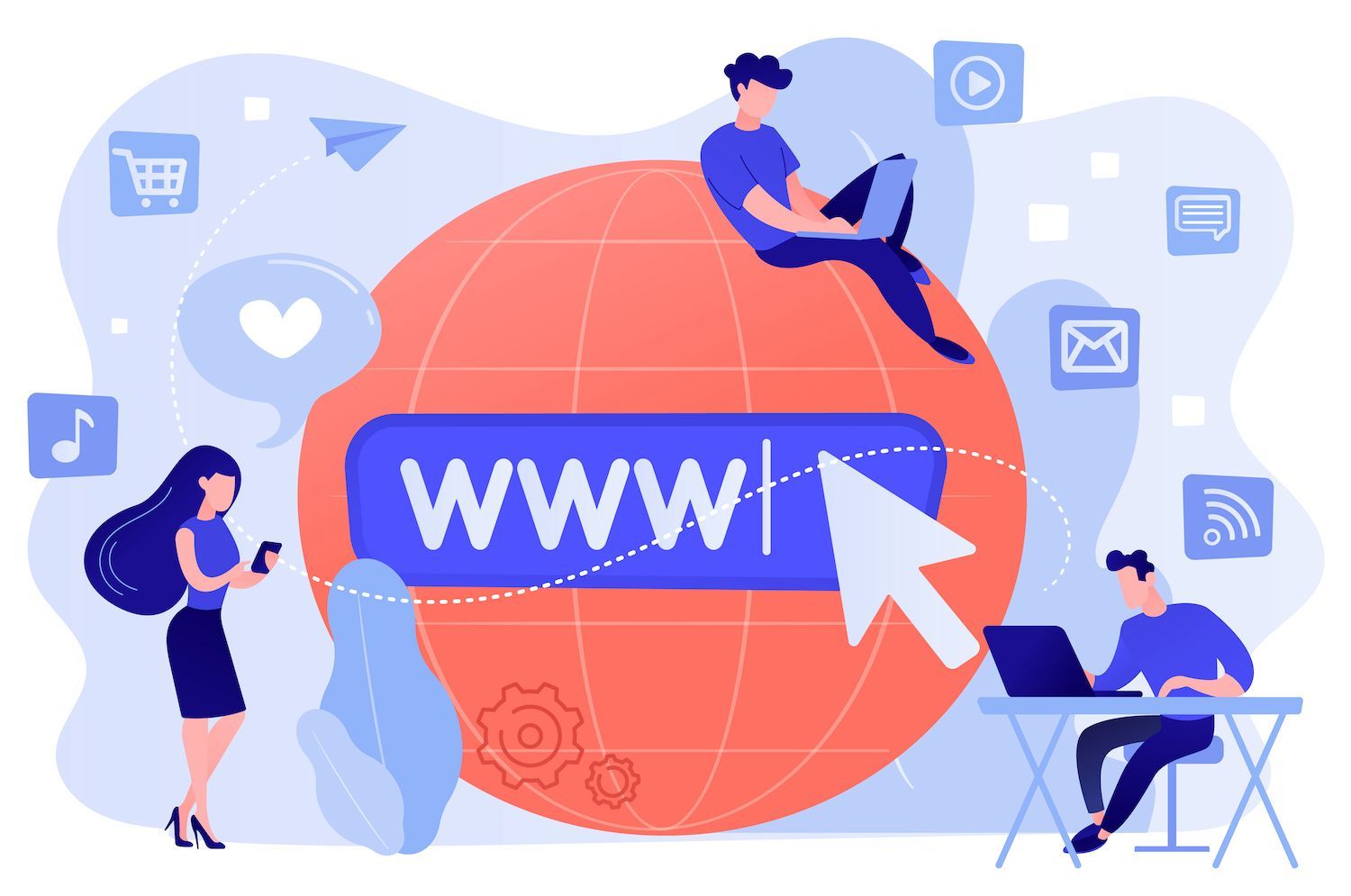
The right-hand side of the panel shows:
- Action icons placed in the row (see further below).
- Watch pane Watch pane allows you to monitor variables using the + icon, then typing the names of them.
- The Breakpoints pane shows an overview of the breakpoints available and allows them to be turned off or activated.
- Scope pane Scope pane shows the state of every module, local and global variables. This is the pane that which you'll most often.
- An Call Stack pane lists the order of calls made to arrive at this level.
The row of icons for action is shown above The icons representing action are paused at breakpoints:
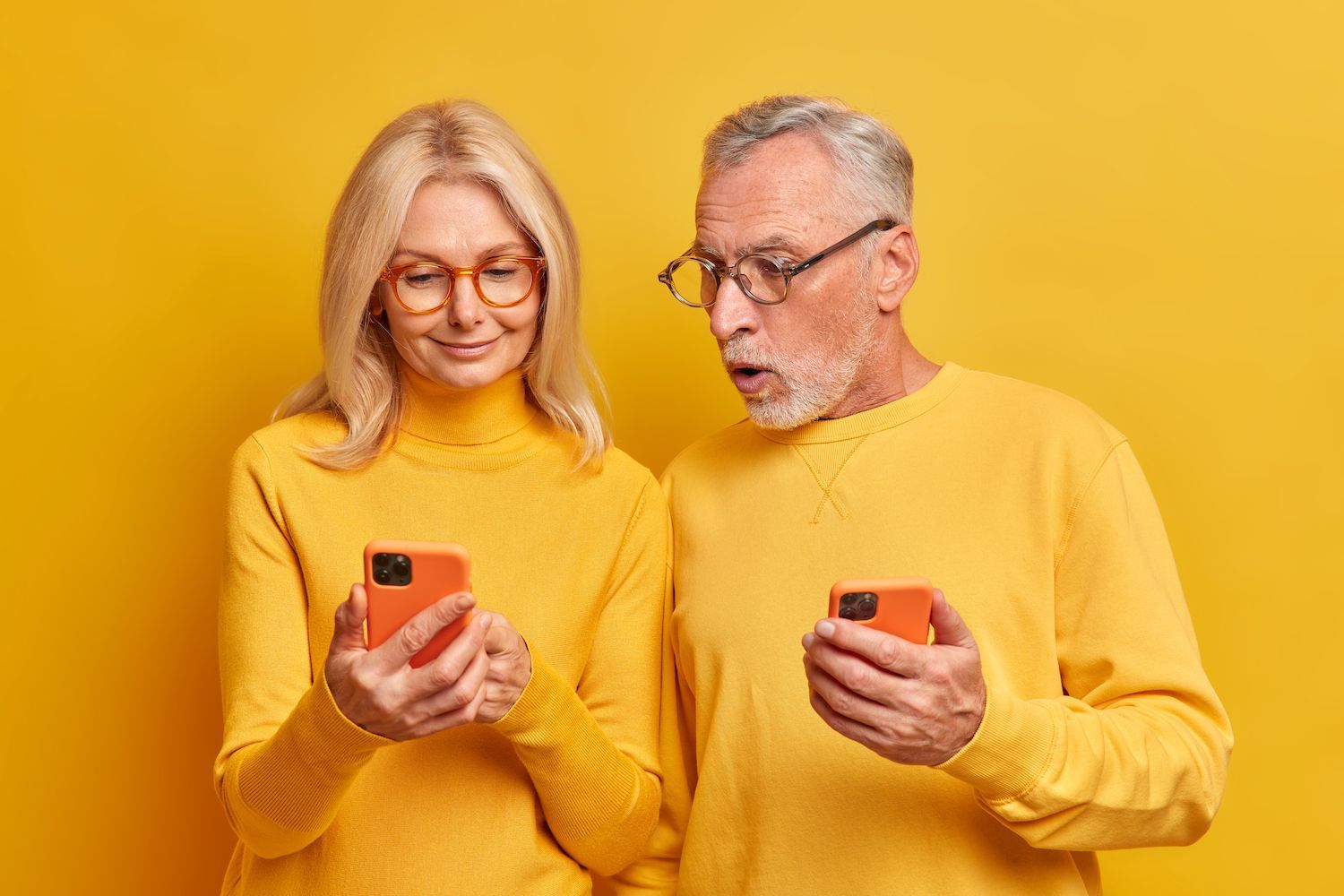
From left to right starting from the left
- resume execution: Continue working until the breakpoint is reached.
- step-over The next step is to execute the command, and remain inside the section of code in use at the moment. Don't dive into the commands it invokes.
- Step into Step into HTML0: Execute the following command, and then move on to the next function as necessary
- eliminate : Continue processing to the end of the operation before returning to the calling command
- step is similar as the step but it does not have the ability to jump into functions that are async.
- disable all breakpoints
- Stops processing in the case of an error Processing is stopped when the error is found.
Conditional Breakpoints
Sometimes, it's essential to have some more influence over the breakpoints. Take a look at a loop which has ran 1,000 times and you're only interested in what happened in the initial one.
for (let i = 0; i
If you prefer not to press to resume execution 999 times by right-clicking the line, choose the option to create an a conditional breakpoint and then define a particular circumstance, for example the number i equals 99
:
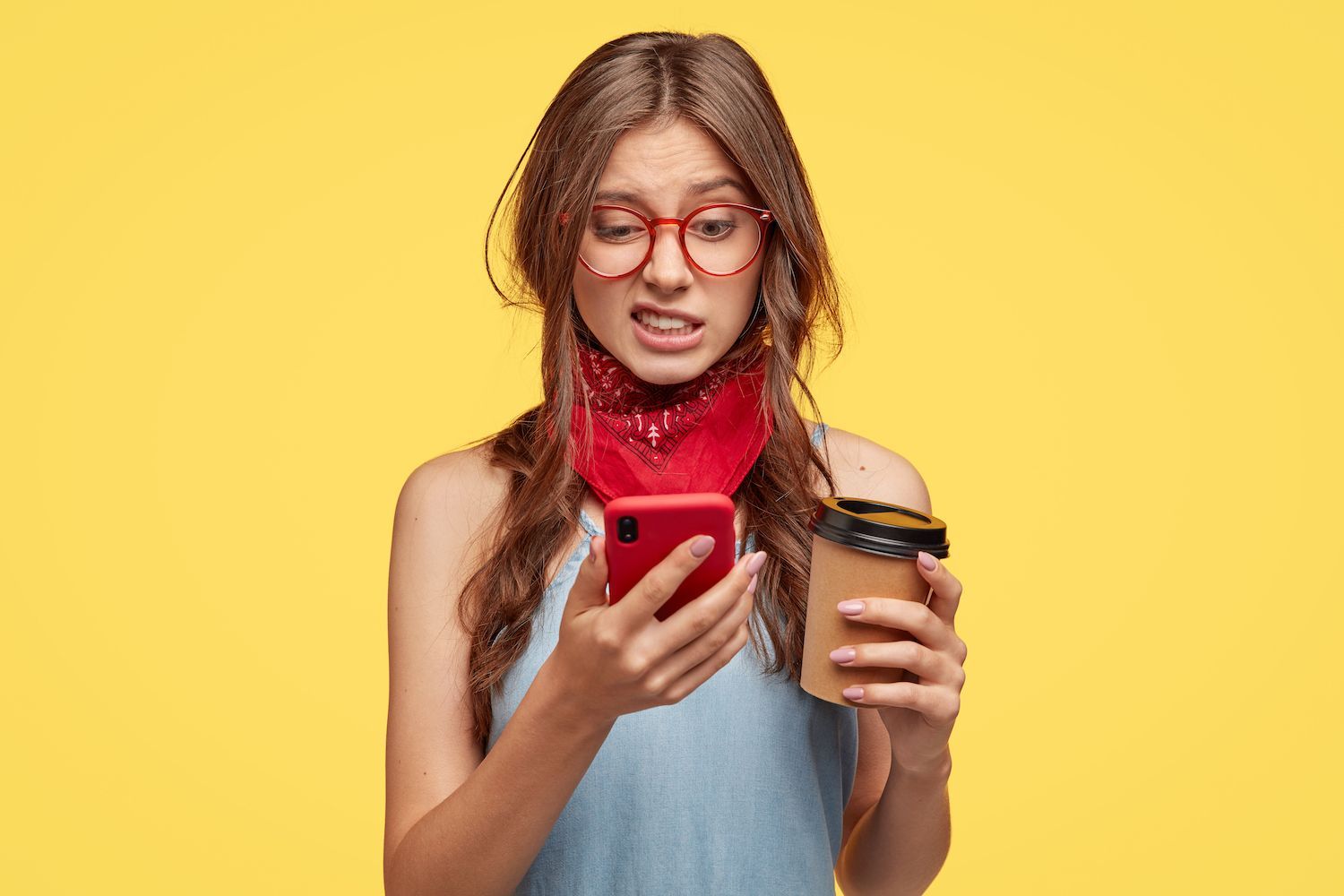
Chrome displays conditional breakpoints as blue, not yellow. This means that the breakpoint will only be active after the completion in the loop.
Log Points
Log points effectively enable you to create console.log() without code! Expressions can be generated whenever the code runs any line. It will however not cease processing, as would a breakpoint.
To create log points, you need to right-click on any line, then select the option to create a log point, and then enter an expression. e.g. "loop counter"loop counter" (i)
:
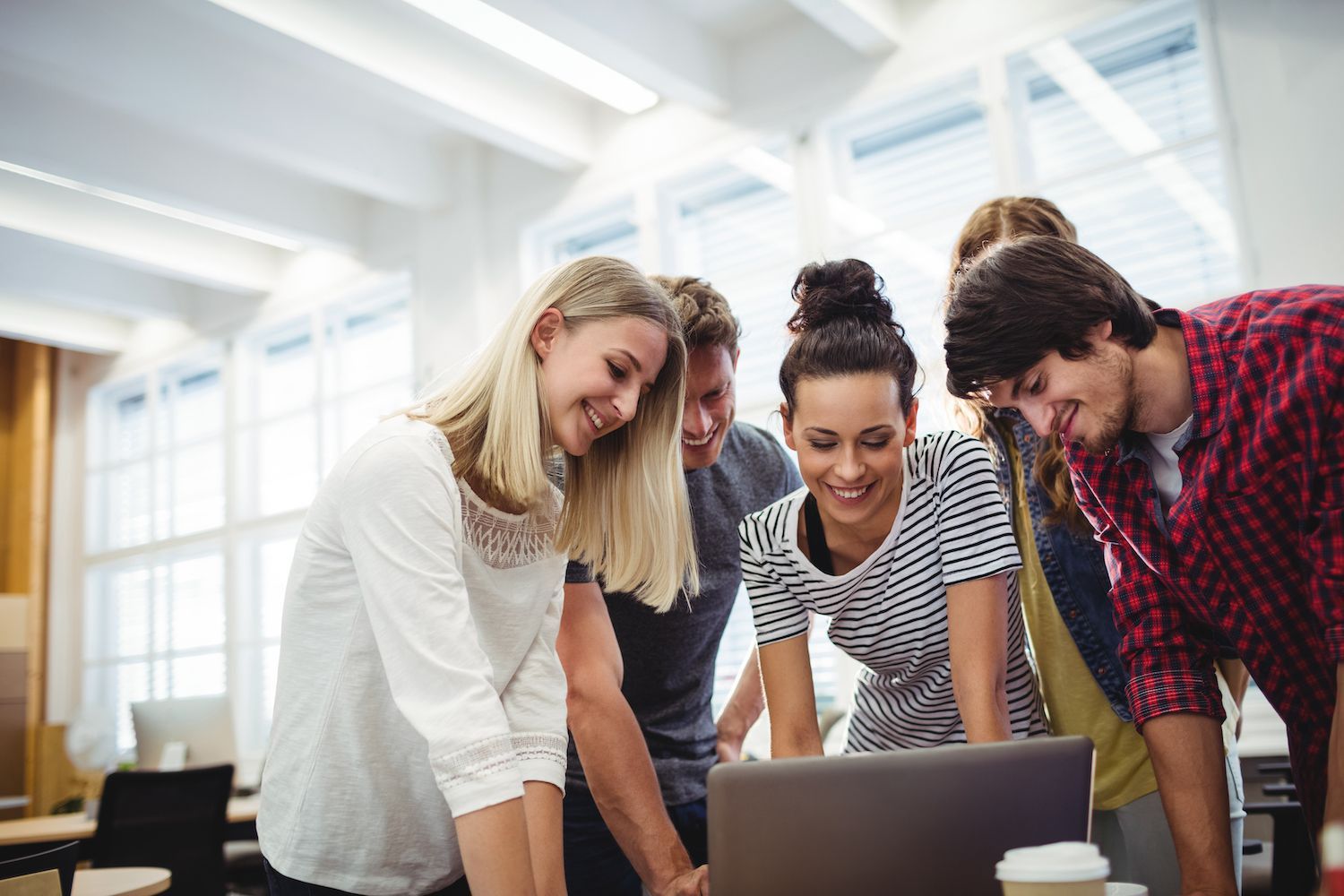
The DevTools console outputs loop counters i.e. for loop counter i.e. 99 in the case above.
Use VS Code to Debug Node.js applications
VS Code is compatible with Node.js and has the integrated debugging tool. A variety of applications are debugged without any setup and the editor will initiate the debugging servers, as well as the client.
Then, open the beginning file (such as index.js) after which, in the the Run and Debuggle pane, then click on the run and debug button, and choose to start to the Node.js environment. Select any line and you will be able to set an breakpoint that is highlighted with a red circle. After that, you can open the app in a browser as before -but VS Code ceases execution after it is at the breakpoint
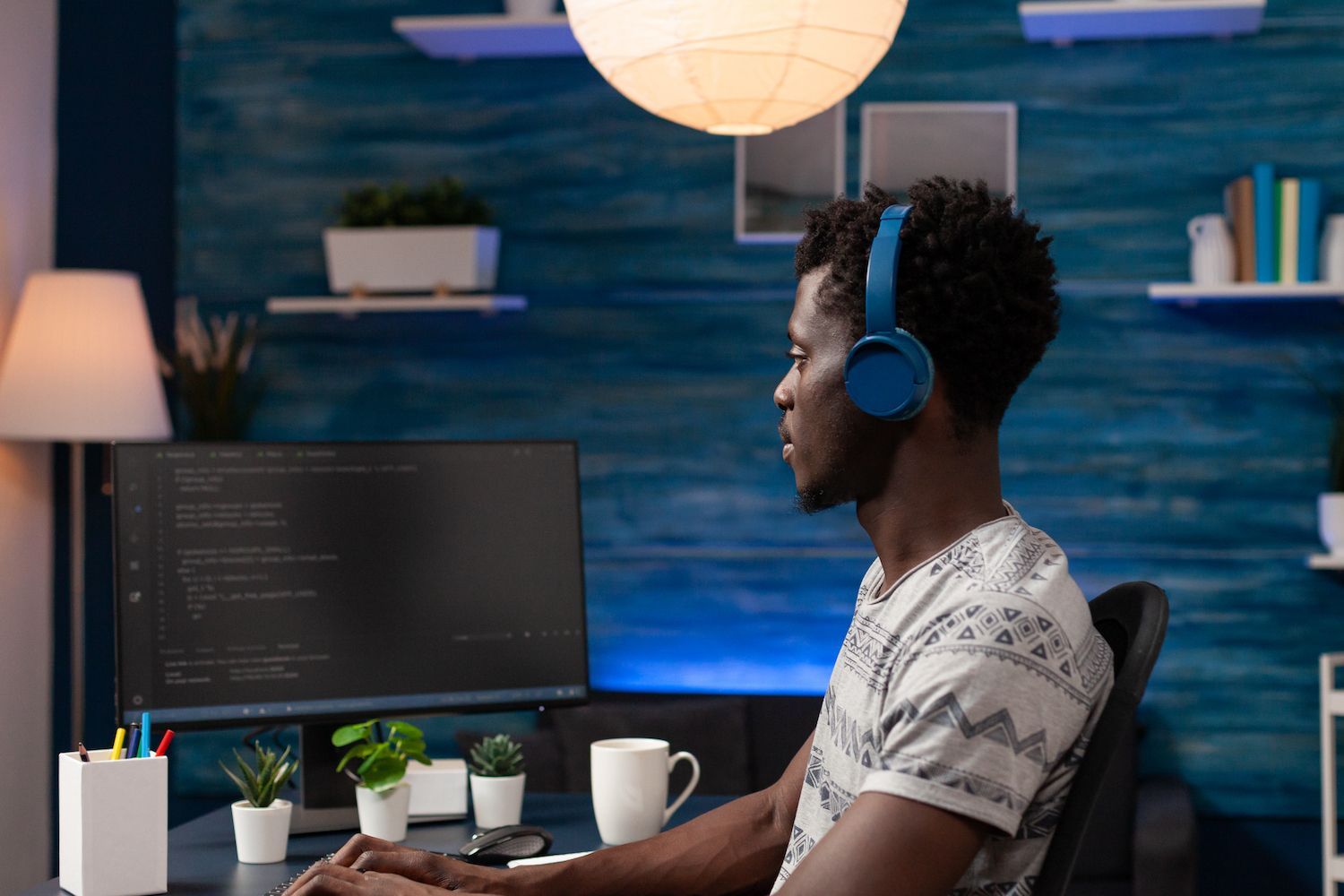
The toolbar for action icons can be used for:
- restart execution to process until the next breakpoint
- Step over step over Follow the steps below, but remain within the functions you're using.Do not run the function it calls
- Step to Step into: Type the command that follows, afterwards, you can jump into the function that it invokes
- Then step out and continue processing until the end of the function before returning to the command line
- Startthe application, and the debugger
- Shut downthe application, as well as the debugger
Similar to Chrome DevTools, it is possible to right-click any line to include conditional breakpoints in addition to log points.
For more information, refer to Debugging in Visual Studio Code.
Advanced Debugging in VS Code Configuration
Furthermore, VS Code configuration may be needed if you plan to debug code on another device, such as such as a virtual machine, or to utilize alternative launch options such as nodemon.
VS Code stores debugging configurations inside an launch.json file that is located inside the .vscode
directory in the project. Start with the run and debugging tab. To create a launch.json file choose to make use of this Node.js environment to create this file. The following example configuration can be found:
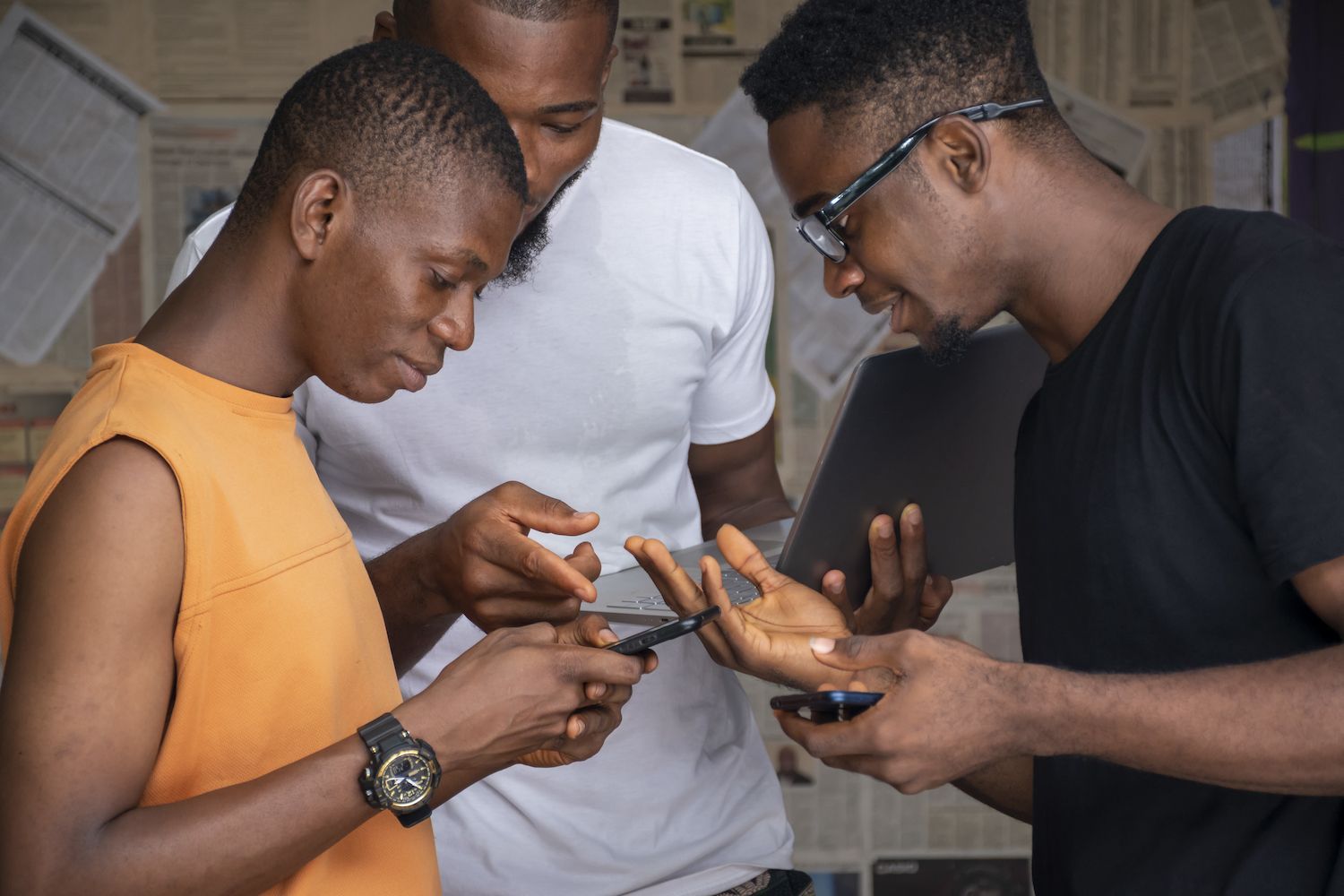
The various configuration settings are available under the "configurations"
array. Click on the Add Configuration... and choose the appropriate option.
An individual Node.js configuration can either:
- The process may be started by itself or
- Attach to the testing Web Socket server, perhaps which is running on a remote device or Docker container.
For example, to define an Nodemon configuration, you must select Node.js Setup: Nodemon and then edit to modify the "program" entry script in the manner that is needed:
// custom configuration "version": "0.2.0", "configurations": [ "console": "integratedTerminal", "internalConsoleOptions": "neverOpen", "name": "nodemon", "program": "$workspaceFolder/index.js", "request": "launch", "restart": true, "runtimeExecutable": "nodemon", "skipFiles": [ "/**" ], "type": "pwa-node" ]
The launch.json
file and the nodemon configuration(the name of the configuration "name") is displayed in the drop-down menu on the right side in the Run and Debug pane. Select the green Run icon to enable the configuration. Start the app using nodemon.
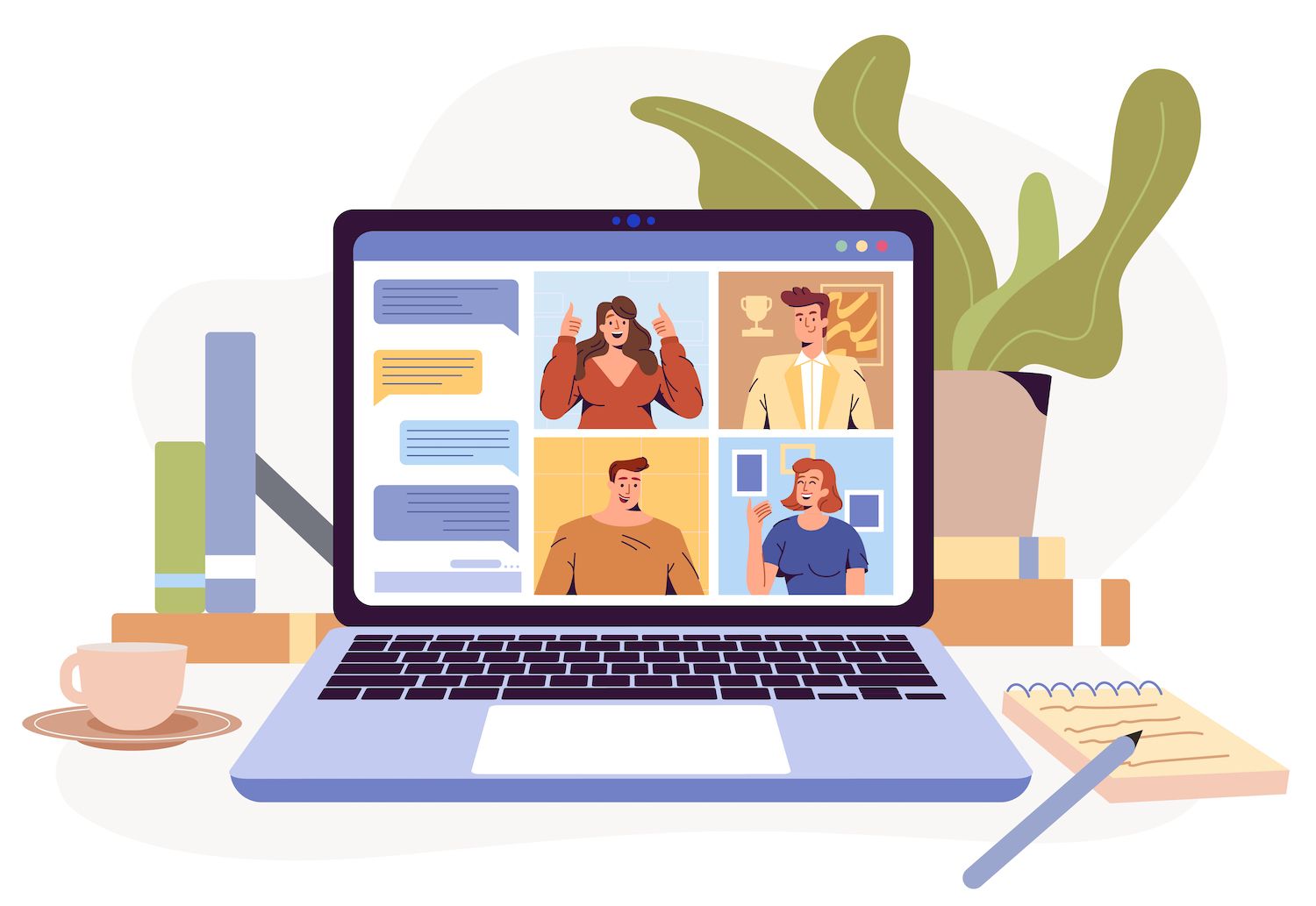
As before, you can build breakpoints, conditional breakpoints as well as logpoints. One of the major differences is that nodemon starts your server when the application's configuration changes.
For further information for more details, please visit the configurations for VS Code Launch.
The following VS Code extensions can also aid you in debugging code that is stored on remote or isolated servers:
- Remote Containers are connected to the applications which are running within Docker containers
- Remote SSH Connect to programs that run on remote servers
- Remote WSL Connect to programs that are run by Windows Subsystem for Linux (WSL). Windows Subsystem for Linux (WSL).
Others Node.js Debugging Options
Its Node.js Debugging Manual provides advice for the various editors available for text and IDEs, which includes Visual Studio, JetBrains WebStorm, Gitpod, and Eclipse. Atom has the node-debug plugin that integrates with an integrated Chrome DevTools debugger into the editor.
When your application has gone live You may want to consider using commercial debugging services such as LogRocket and Sentry.io, which allows you to record and replay error messages received by real-world clients.
Summary
Utilize any instrument you are able to use in identifying an problem. It's fine to use console.log() for console.log() to speed up bug hunting However, Chrome DebTools and VS Code may be preferable for more complex problems. These tools can help you create more secure software and will help you spend less time attempting to solve issues.
What Node.js practices is your most reliable method? Please share your thoughts in the comments below!
Make it easier to save time, money and improve site performance by:
- Help is available immediately from WordPress experts in hosting all hours of the day.
- Cloudflare Enterprise integration.
- Reaching a global audience with 29 data centers across the globe.
- Optimization through the integrated Application to measure the performance.
This post was posted on here